To find files modified in the last hour using PowerShell, you can use the Get-ChildItem cmdlet along with the Where-Object cmdlet to filter the result based on the LastWriteTime property.
The following method shows how to use it with syntax.
Method 1: Find files modified in the last hour
# specify the path to the folder
$folderPath = "C:\temp\log\"
# get the last hour
$lastHour = (Get-Date).AddHours(-1)
# Retrieve files and find files modified in the last hour
Get-ChildItem -Path $folderPath -Recurse | Where-Object {$_.LastWriteTime -gt $lastHour}
This example will list files modified in the last hour.
Method 2: Find files modified in the last 24 hours
# specify the path to the folder
$folderPath = "C:\temp\log\"
# get the last 24 hour
$last24Hour = (Get-Date).AddHours(-24)
# Retrieve files and find files modified in the last 24 hour
Get-ChildItem -Path $folderPath -Recurse | Where-Object {$_.LastWriteTime -gt $last24Hour}
This example will find files modified in the last 24 hours.
The following examples show how to use these methods.
Find Files Modified in the Last Hour in PowerShell
Use the Get-ChildItem cmdlet in PowerShell to retrieve the list of files from the specified path and filter based on the LastWriteTime property of the file to list files modified in the last hour.
# specify the path to the folder $folderPath = "C:\temp\log\" # get the last hour $lastHour = (Get-Date).AddHours(-1) # Retrieve files and find files modified in the last hour Get-ChildItem -Path $folderPath -Recurse | Where-Object {$_.LastWriteTime -gt $lastHour}
Output:
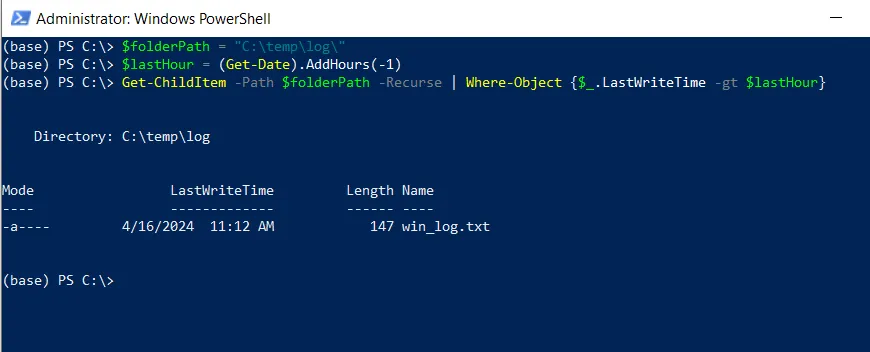
The Get-ChildItem cmdlet uses the -Path and -Recurse parameters to specify the $folderPath to retrieve all files recursively from the directory and pipes them to the Where-Object cmdlet.
Finally, the Where-Object filters the result by comparing the LastWriteTime of the file with the last hour. It keeps only those files where the LastWriteTime property is greater than the $lastHour.
After executing the script, it returns a list of all files in the specified directory and its subdirectories that have been modified within the last hour.
Find Files Modified in the Last 24 Hours in PowerShell
To find files modified in the last 24 hours in the directory, use the Get-ChildItem with the Where-Object cmdlets to retrieve files and filter based on the LastWriteTime of the file greater than the last 24 hours.
# specify the path to the folder $folderPath = "C:\temp\log\" # get the last 24 hour $last24Hour = (Get-Date).AddHours(-24) # Retrieve files and find files modified in the last 24 hour Get-ChildItem -Path $folderPath -Recurse | Where-Object {$_.LastWriteTime -gt $last24Hour}
Output:
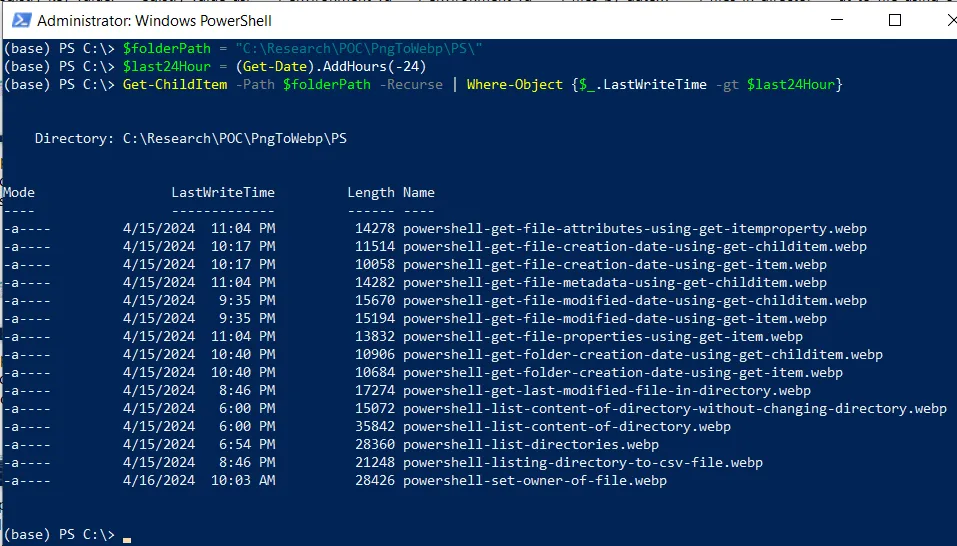
The Get-ChildItem cmdlet uses the -Path and -Recurse parameters to specify the $folderPath to retrieve all files recursively from the directory and pipes them to the Where-Object cmdlet.
Finally, the Where-Object filters the result by comparing the LastWriteTime of the file with the last 24 hours. It keeps only those files where the LastWriteTime property is greater than the $last24Hour.
After executing the script, it returns a list of all files in the specified directory and its subdirectories that have been modified within the last 24 hours.
You can modify the time interval by changing the value passed to the AddHours() method to search for files modified in the different time intervals.
Conclusion
I hope the above article on how to get a list of files modified in the last hour or the last certain hours in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.