To use multiple filters using the Get-ChildItem cmdlet in PowerShell, you can use the following methods.
Method 1: Using the -Filter parameter with multiple filters
$filters = "*.txt","*.log"
$files = foreach ($filter in $filters) {
Get-ChildItem -Path $folderPath -Filter $filter
}
This example will return files with extension .txt and .log from the current directory.
Method 3: Using Where-Object to filter results
Get-ChildItem | Where-Object {$_.Extension -in ".txt", ".log"}
This example will return files with extension .txt and .log in the current directory based on specified search criteria.
The following example shows how you can use these methods.
How to Use Get-ChildItem cmdlet with Multiple Filters in PowerShell
To use the Get-ChildItem cmdlet with multiple filters in PowerShell, you can specify an array of filters.
# specify the folder path $folderPath = "C:\temp\log\" $filters = "*.txt","*.log" # Apply multiple filters with Get-ChildItem $files = foreach ($filter in $filters) { Get-ChildItem -Path $folderPath -Filter $filter } Write-Output $files
Output:
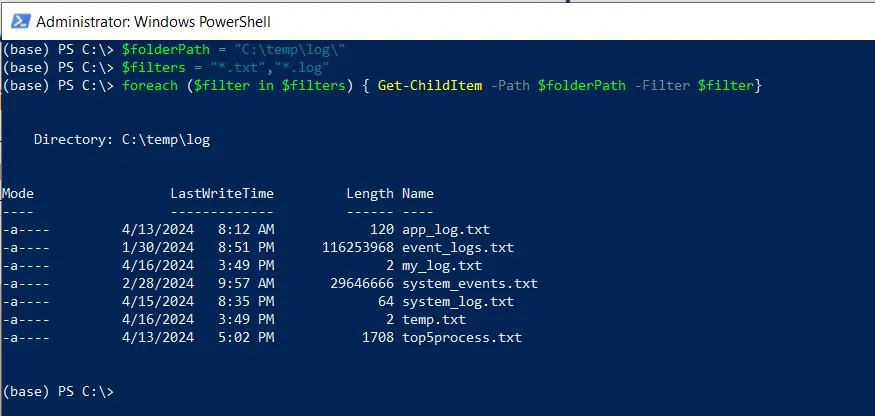
In this script, we define a variable $folderPath that contains the directory path.
We then use the Get-ChildItem cmdlet with multiple filters “.txt”, and “.log” specified with the -Filter parameter to filter the result.
It will retrieve all files that match extension .txt or .log and store them in the $files variable.
Finally, we use the Write-Output cmdlet to output the files.
How to Use Get-ChildItem with Where-Object to Filter Results
You can use the Get-ChildItem cmdlet with the Where-Object cmdlet in PowerShell to apply the multiple filters and filter the result.
# specify the folder path $folderPath = "C:\temp\log\" # Apply multiple filters with Get-ChildItem $files = Get-ChildItem -Path $folderPath | Where-Object {$_.Extension -in ".txt", ".log"} Write-Output $files
Output:
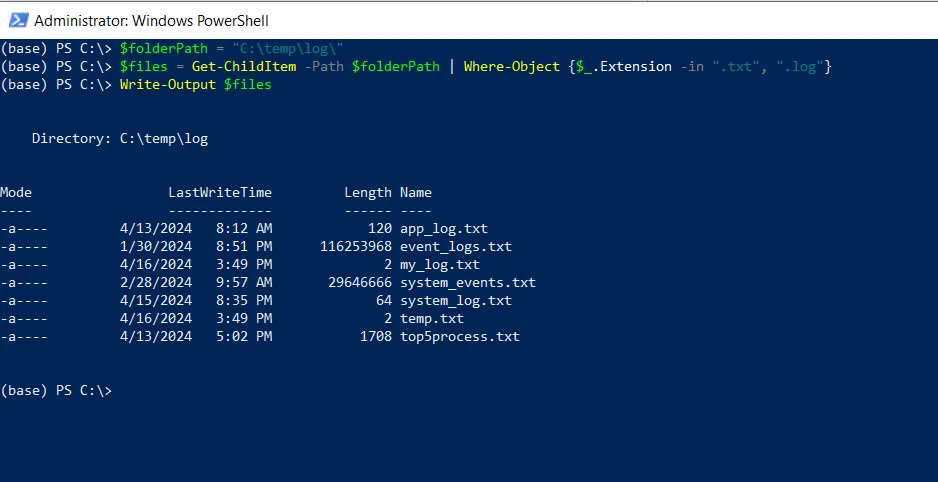
In this script, we define a variable $folderPath to store the directory path.
We then use the Get-ChildItem cmdlet in PowerShell to retrieve all files and pipe them to the Where-Object cmdlet to apply multiple filters.
It retrieves those files that match the .txt or .log extension specified in the criteria and stores the result in the $files variable.
Finally, we use the Write-Outut cmdlet to output the files.
Conclusion
I hope the above article on using the Get-ChildItem cmdlet with multiple filters in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.