To get a file modified date using PowerShell, you can use the Get-Item or Get-ChildItem cmdlets to retrieve file information and then access the LastWriteTime property.
The following methods show how you can do it.
Method 1: Get the file modified date using the Get-Item cmdlet
# Specify the file path
$filePath = "C:\temp\log\system_log.txt"
# Retrieve file information and get file modified date
$fileModifiedDate = (Get-Item -Path $filePath).LastWriteTime
Write-Output $fileModifiedDate
This example returns the file modified date using the Get-Item cmdlet.
Method 2: Get the file modified date using the Get-ChildItem cmdlet
# Specify the file path
$filePath = "C:\temp\log\system_log.txt"
# Retrieve file information and get file modified date
$fileModifiedDate = (Get-ChildItem -Path $filePath -File).LastWriteTime
Write-Output $fileModifiedDate
This example will get the file modified date using the Get-ChildItem cmdlet in PowerShell.
The following examples show how to use these methods.
Get File Modified Date in PowerShell Using Get-Item
To get the file modified date, use the Get-Item cmdlet to retrieve file information and then access the LastWriteTime property. The LastWriteTime property returns the last modified date of the file.
# Specify the file path $filePath = "C:\temp\log\system_log.txt" # Retrieve file information and get file modified date $fileModifiedDate = (Get-Item -Path $filePath).LastWriteTime Write-Output $fileModifiedDate
Output:
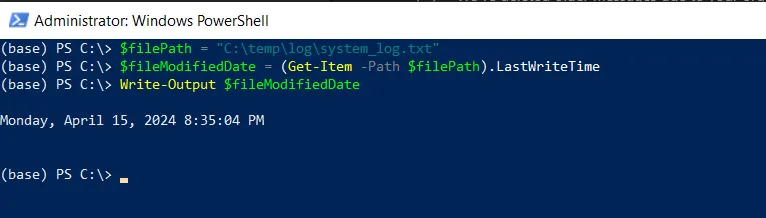
This example uses the Get-Item command to retrieve the file information specified by the $filePath variable and access the LastWriteTime property to get the file’s modified date.
Finally, the Write-Output command prints the last modified date of the file.
Get File Modified Date Using the Get-ChildItem in PowerShell
Another way to get a file modified date is by using the Get-ChildItem cmdlet and accessing the LastWriteTime property.
# Specify the file path $filePath = "C:\temp\log\system_log.txt" # Retrieve file information and get file modified date $fileModifiedDate = (Get-ChildItem -Path $filePath -File).LastWriteTime Write-Output $fileModifiedDate
Output:
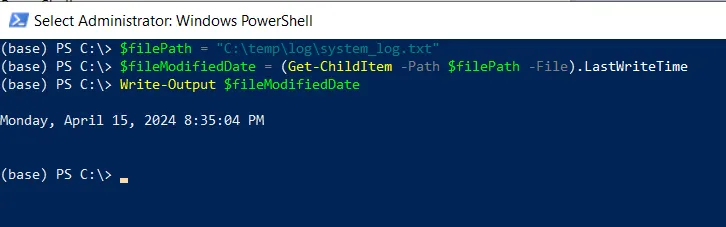
In this PowerShell script, the Get-ChildItem command retrieves the file information for the $filePath and accesses the LastWriteTime property of the file.
Finally, the Write-Output command outputs the last modified date of the file.
Conclusion
I hope the above article on getting the last modified date of the file using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.