In PowerShell, you can compute the MD5 hash value of a string or file using the Get-FileHash cmdlet. The Get-FileHash command uses the Algorithm parameter to specify the hash algorithm you want to use.
The following methods can be used to calculate the MD5 hash of a string or file in PowerShell.
Method 1: Calculate MD5 of a string
# Define the string
$someString = "This is some text for MD5 hash"
# Comvert the string to bytes
$stringBytes = [System.Text.Encoding]::UTF8.GetBytes($someString)
# Create a MD5 hash object
$md5 = [System.Security.Cryptography.HashAlgorithm]::Create("MD5")
# Compute the MD5 hash
$hashBytes = $md5.ComputeHash($stringBytes)
# Convert the hash bytes to a hexadecimal string
$hashString = [System.BitConverter]::ToString($hashBytes)
$hashString = $hashString.Replace("-", "")
# Output the MD5 hash of a string
Write-Output $hashString
This example calculates the MD5 hash of a string.
Method 2: Calculate the MD5 of a File
# Define the file path
$filePath = "C:\temp\log\system_log.txt"
# Get the file hash using the MD5 algorithm
$fileHash = Get-FileHash -Path $fileHash -Algorithm MD5
# Output the hash of a file
Write-Output $fileHash.Hash
This example calculates the MD5 of a file.
The following examples show how to use these methods.
Get MD5 Hash of a String in PowerShell
To get the MD5 hash of a string in PowerShell, you can use the [System.Security.Cryptography.MD5] .NET class.
# Define the string $someString = "This is some text for MD5 hash" # Comvert the string to bytes $stringBytes = [System.Text.Encoding]::UTF8.GetBytes($someString) # Create a MD5 hash object $md5 = [System.Security.Cryptography.HashAlgorithm]::Create("MD5") # Compute the MD5 hash $hashBytes = $md5.ComputeHash($stringBytes) # Convert the hash bytes to a hexadecimal string $hashString = [System.BitConverter]::ToString($hashBytes) $hashString = $hashString.Replace("-", "") # Output the MD5 hash of a string Write-Output $hashString
Output:
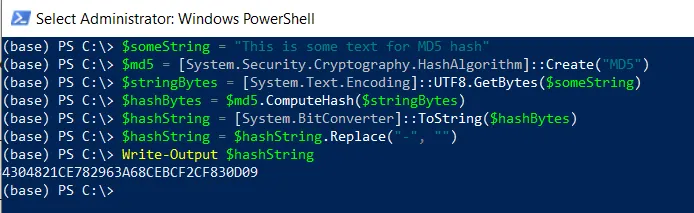
In this script, the $someString variable holds the string data. We convert the string into the bytes and store it into the $stringBytes variable.
[System.Security.Cryptography.MD5]::Create() method creates an instance of the MD5 hash algorithm. The ComputeHash() method computes the hash value of the input byte array. Later, we convert the hash bytes to a hexadecimal string.
Finally, the Write-Output cmdlet outputs the MD5 hash of a string to the console.
After running the script, it calculates the MD5 hash of the specified string “This is some text for MD5 hash” and displays it on the console.
Get MD5 Hash of a File in PowerShell
To get the MD5 hash of a file in PowerShell, you can use the Get-FileHash cmdlet with the MD5 Algorithm.
# Define the file path $filePath = "C:\temp\log\system_log.txt" # Get the file hash using the MD5 algorithm $fileHash = Get-FileHash -Path $fileHash -Algorithm MD5 # Output the hash of a file Write-Output $fileHash.Hash
Output:
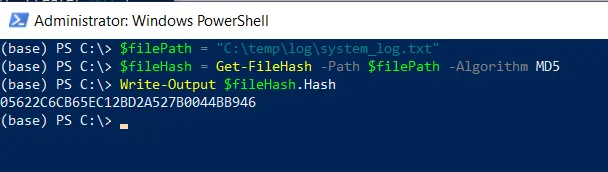
In the above PowerShell script, the $filePath variable stores the path to the file. The Get-FileHash cmdlet is used to compute the hash value of a file $filePath using the MD5 algorithm.
Finally, it outputs the MD5 file hash to the console.
After running the script, it calculates the MD5 hash of the file “system_log.txt” and displays it on the console.
Conclusion
I hope the above article on getting the MD5 hash value of a string or file in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.