To compare file dates in PowerShell, you can use the Get-Item
or Get-ChildItem
cmdlets to retrieve file information and compare their LastWriteTime
property, which indicates when the file was last time modified.
The following method shows how you can do it with syntax.
Method 1: Compare file dates
$file1 = "C:\temp\log\test1.txt"
$file2 = "C:\temp\log\test2.txt"
$file1Info = Get-Item $file1
$file2Info = Get-Item $file2
If($file1Info.LastWriteTime -gt $file2Info.LastWriteTime) {
Write-Output "$file1 is newer than $file2"
}
else if($file1Info.LastWriteTime -gt $file2Info.LastWriteTime) {
Write-Output "$file1 is older than $file2"
}
else {
Write-Output "$file1 and $file2 have the same modification date"
}
This example will compare two file dates and output which file is newer, older, or if they have the same modification date.
The following example shows how you can use this method to compare file dates in PowerShell.
Compare File Dates in PowerShell
You can compare the last modified dates of two files to determine which one is newer, older, or if they are the same.
Here’s an example of how to compare the last modification dates of two files in PowerShell.
# Define the paths to the two files $file1 = "C:\temp\log\system_log.txt" $file2 = "C:\temp\log\system_events.txt" # retrieve the files information $file1Info = Get-Item $file1 $file2Info = Get-Item $file2 # Compare the LastWriteTime of the files If($file1Info.LastWriteTime -gt $file2Info.LastWriteTime) { Write-Output "$file1 is newer than $file2" } elseif($file1Info.LastWriteTime -lt $file2Info.LastWriteTime) { Write-Output "$file1 is older than $file2" } else { Write-Output "$file1 and $file2 have the same modification date" }
Output:
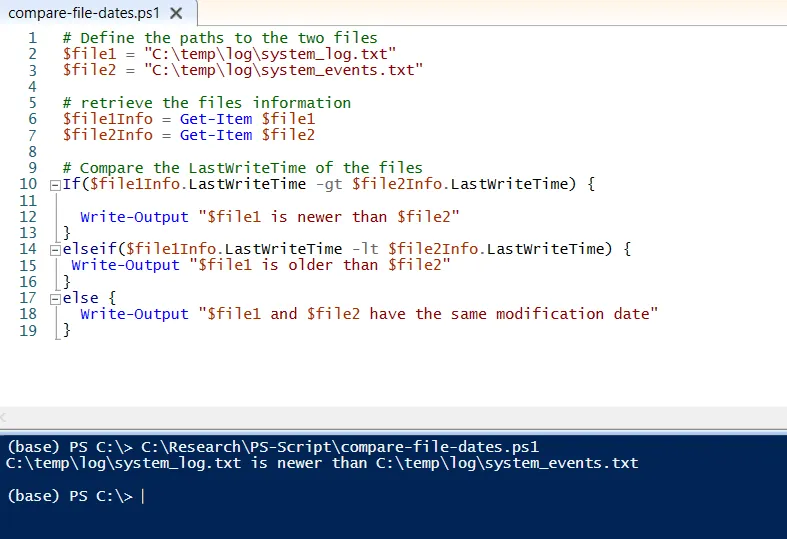
In this example, we define the $file1
and $file2
variables and assign them the path to the files that we want to compare.
We then retrieved the file information using the Get-Item cmdlet and stored it in the $file1Info
and $file2Info
variables.
Finally, we compare the file’s LastWriteTime
property using the -gt
(greater than) and -lt
(less than) operators.
After running the PowerShell script, it compares two file dates and outputs which file is newer, older, or if they have the same last modification date.
In the above example, $file1
has Sunday, April 21, 2024 9:45:17 AM and $file2
has Wednesday, February 28, 2024 9:57:34 AM last modified dates. After comparing using the -gt or -lt operators, it outputs “C:\temp\log\system_log.txt is newer than C:\temp\log\system_events.txt“
Conclusion
I hope the above article on comparing two file dates in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.