To convert a date to epoch time in PowerShell, you can use the Get-Date cmdlet to get the date and time and subtract it from the Unix epoch start time (January 1, 1970).
The following methods show how you can do it with syntax.
Method 1: Convert date to Unix epoch time
$date = Get-Date "2022-04-28 12:00:00"
$epochTime = [Math]::Round((New-TimeSpan -Start "1970-01-01" -End $date).TotalSeconds)
This example will return the number of seconds that have elapsed since January 1, 1970.
Method 2: Convert date to epoch time with -UFormat parameter
$currentDate = (Get-Date).ToString("yyyy-MM-dd HH:mm:ss")
[int]::Parse((Get-Date $currentDate -UFormat %s))
This example will return the Unix epoch time.
The following examples show how to use these methods to convert date to epoch time in PowerShell.
Convert Date to Unix Epoch Time in PowerShell
You can convert date to Unix epoch time, which represents the number of seconds that have elapsed since January 1, 1970. Here’s how you can do it in PowerShell script.
# Define the date $date = Get-Date "2024-04-01 10:00:00" # Convert the date to Unix epoch time $epochTime = [Math]::Round((New-TimeSpan -Start "1970-01-01" -End $date).TotalSeconds) # Output the Unix epoch time Write-Output "Unix Epoch Time: $epochTime"
Output:
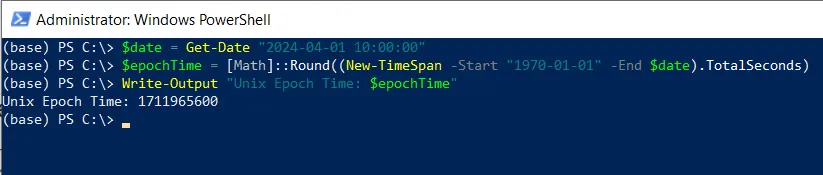
In this example, we define a $date
variable that contains the date (2024-04-01 10:00:00) you want to convert to epoch time.
New-TimeSpan -Start "1970-01-01" -End $date
calculates the time difference between the Unix epoch (January 1, 1970) and the specified date $date.
.TotalSeconds
retrieves the total number of seconds in the time difference. We the use the [Math]::Round() function to round the total number of seconds to the nearest whole number and store the result in the $epochTime
variable.
Finally, we output the Unix epoch time $epochTime
using the Write-Output
cmdlet in PowerShell.
In this example, converting the date 2024-04-01 10:00:00 to epoch time output 1711965600 total seconds.
Convert Date to Epoch Time with -UFormat Parameter in PowerShell
You can convert date to epoch time by using the Get-Date
cmdlet with the -UFormat %s
parameter. Here’s how you can do it in PowerShell script.
# get the current date in specific format $currentDate = (Get-Date).ToString("yyyy-MM-dd HH:mm:ss") # Convert the date to epoch time using -UFormat %s [int]::Parse((Get-Date $currentDate -UFormat %s))
Output:
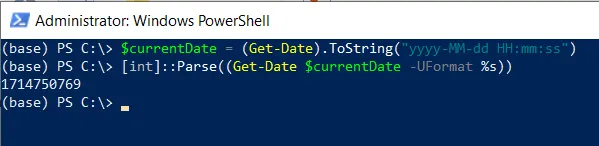
In this example, the $currentDate variable contains the today’s date retrieved using the Get-Date
cmdlet in PowerShell.
We then use the Get-Date cmdlet with -UFormat %s parameter to convert the date to Unix epoch time in PowerShell.
After running the PowerShell script, it output the total number of seconds that elapsed since January 1, 1970, in this case, it returns 1714750769 total seconds.
Conclusion
I hope the above article on converting the date to Unix epoch time in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.