To subtract dates in PowerShell, you can subtract one datetime object from another, it returns a TimeSpan object, which represents the difference between the two dates.
The following method shows how you can do it with syntax.
Method 1: Subtract dates in PowerShell
$date1 = Get-Date "2024-01-04"
$date2 = Get-Date "2024-01-05"
$date2 - $date1
This example will subtract the dates in PowerShell and return the date difference between the two dates as a TimeSpan
object.
The following examples show how to use this method to subtract dates in PowerShell.
Subtract Dates to Get Date Difference in Months in PowerShell
To get date differences in months in PowerShell, here’s how you can do it.
# define the two dates in format ( yyyy-MM-dd) $date1 = Get-Date "2024-01-04" $date2 = Get-Date "2024-03-05" # Calculate the difference in months $monthsDifference = ($date2.Year - $date1.Year) * 12 + ($date2.Month - $date1.Month) if ($date2.Day -lt $date1.Day) { $monthsDifference-- } Write-Output "Difference between dates in months: $monthsDifference"
Output:
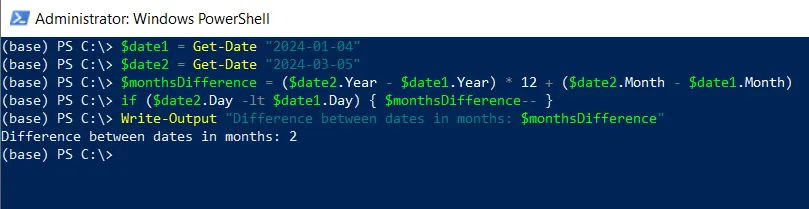
In this example, we define two dates $date1
and $date
in the format (yyy-MM-dd).
We then calculate the difference between $date1
and $date2
. If the $date2
is in a later month but earlier in the current month than $date1
, we adjust the difference by subtracting 1.
Finally, after running the script outputs the difference in months.
Subtract Dates to Get Date Difference in Hours in PowerShell
To get the date difference in days in PowerShell, you can subtract the datetime object from another date, it returns the TimeSpan
object.
You can then access the Hours property of the TimeSpan
object to get the difference in hours.
# define two dates in format (yyyy-MM-dd) $date1 = Get-Date "2024-01-04" $date2 = Get-Date "2024-01-10" # Calculate the difference in dates $diff = $date2 - $date1 # Output the date difference in hours Write-Output "Difference in Hours: $($diff.TotalHours)"
Output:
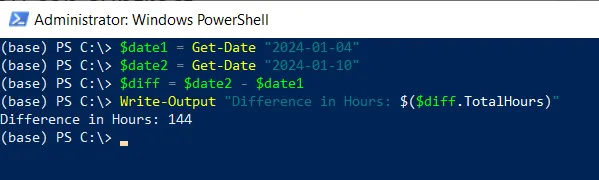
In this example, we define two dates $date1
and $date2
in format (yyyy-MM-dd). We then calculate the difference between the two dates and store the resulting TimeSpan
object in the $diff
variable.
Next, we can access the TotalHours
property of TimeSpan
object to get the difference in hours.
After running the PowerShell script, it outputs the difference in hours for the given dates.
Subtract Dates to Get Date Difference in Minutes in PowerShell
To get the date difference in minutes in PowerShell, here’s how you can do it.
# define two dates in format (yyyy-MM-dd) $date1 = Get-Date "2024-01-04" $date2 = Get-Date "2024-01-10" # Calculate the difference in dates $diff = $date2 - $date1 # Output the date difference in minutes Write-Output "Difference in minutes: $($diff.TotalMinutes)"
Output:
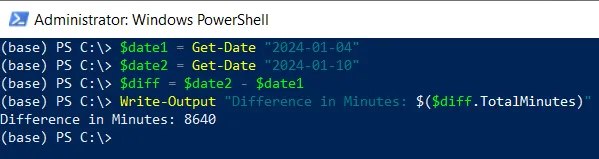
In this example, we define two dates $date1
and $date2
. We then subtract dates to calculate the difference in dates and store the resulting TimeSpan
object in the $diff
variable.
Finally, we can access the TotalHours
property of the TimeSpan
object to get the date difference in hours.
Conclusion
I hope the above article on subtracting dates in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.