To create a file with the current date in its name in PowerShell, you can use the New-Item cmdlet to create a file if it doesn’t exist and the Get-Date cmdlet to retrieve the current date and format it.
The following method shows how you can do it.
Method 1: Create a file with the current date in its name
# specify the file path
$filePath = "C:\temp\log\my_log.txt"
# Get the current date and format it as dd-MM-yyyy
$currentDate = Get-Date -Format "dd-MM-yyyy"
# append the current date to the file name
$filePath = $filePath + "_" + $currentDate
# create the new file
New-Item -Path $filePath -ItemType File
This example will create a new file if it doesn’t exist.
Create a File with the Current Date in the Name
You can use the New-Item cmdlet in PowerShell to create a new file with its -ItemType parameter set to the “File“. The command checks if the file exists and creates if not.
# specify the file path $filePath = "C:\temp\log\my_log.txt" # Get the current date and format it as dd-MM-yyyy $currentDate = Get-Date -Format "dd-MM-yyyy" # append the current date to the file name $filePath = $filePath + "_" + $currentDate # create the new file New-Item -Path $filePath -ItemType File
Output:
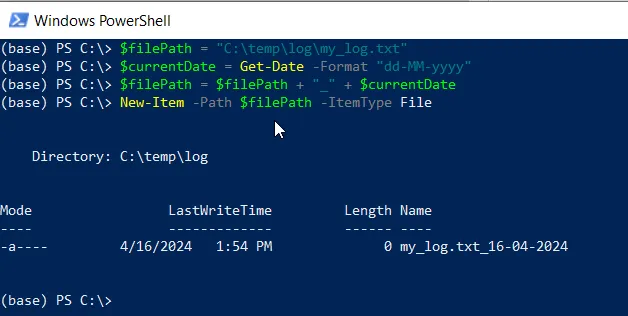
In this PowerShell script, the $filePath variable stores the path to the file you want to create. The $currentDate variable stores the current date in the format “dd-MM-yyyy“. It then appends the current date to the $filePath variable.
Finally, the New-Item command creates a new file at the specified location $filePath.
Conclusion
I hope the above article on creating a new file with the current date in its name in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.