There are several ways to create a file in PowerShell.
The following methods show you can do it with syntax.
Method 1: Using New-Item
New-Item -Path "C:\temp\log\my_log.txt" -ItemType File
This example will create a new file my_log.txt.
Method 2: Using Out-File
"This is some text in file" | Out-File -FilePath "C:\temp\log\my_log.txt"
This example will output the content to the new file named my_log.txt.
Method 3: Using Set-Content
Set-Content -Path "C:\temp\log\my_log.txt" -Value "This is some text in file"
This example will write the content to the file named my_log.txt. It will create a file if it does not exist otherwise it will append the text to the my_log.txt file.
Method 4: Using Add-Content
"This is some text in file" | Add-Content -Path "C:\temp\log\my_log.txt"
This example will add the content to the my_log.txt file.
Method 5: Using FileStream in .NET
$content = "This is some text in file"
$stream = [System.IO.File]::Create("C:\temp\log\my_log.txt")
$stream.Write([System.Text.Encoding]::UTF8.GetBytes($content))
$stream.Close()
This example will create a new file at the specified location, write the specified $content to it, and then close the file stream.
The following examples show how to use these methods.
Create a File Using New-Item in PowerShell
To create a file in PowerShell, you can use the New-Item cmdlet with the -ItemType parameter set to “File“.
The following example shows how to do it with syntax.
New-Item -Path "C:\temp\log\my_log.txt" -ItemType File
Output:
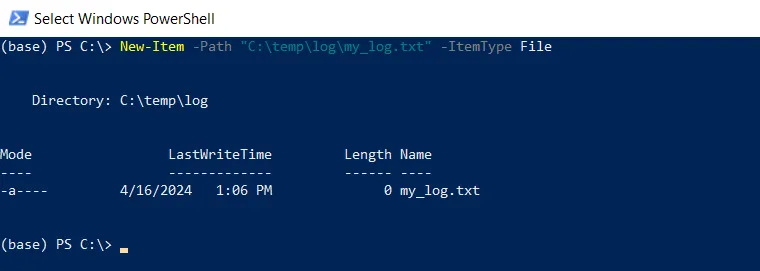
This PowerShell script creates a new file named “my_log.txt” at the specified location.
Create a File Using Out-File in PowerShell
You can use the Out-File cmdlet in PowerShell to create a file. If the file doesn’t exist, it will be created.
"This is some text in file" | Out-File -FilePath "C:\temp\log\my_log.txt"
Output:
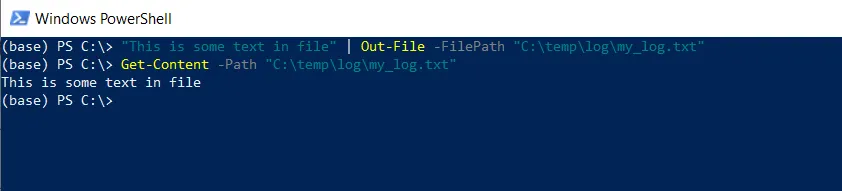
This PowerShell script creates a new file named “my_log.txt” in the “C:\temp\log” directory and writes “This is some text in file” to it.
Create a File Using Set-Content in PowerShell
Another way to create a file and write content to the file is by using the Set-Content cmdlet.
Set-Content -Path "C:\temp\log\my_log.txt" -Value "This is some text in file"
Output:

This PowerShell script creates a new file named “my_log.txt” in the “C:\temp\log\” directory and writes the “This is some text in file“.
Create a File Using Add-Content in PowerShell
You can use the Add-Content cmdlet in PowerShell to create a new file and add content to the file.
"This is some text in file" | Add-Content -Path "C:\temp\log\my_log.txt"
Output:
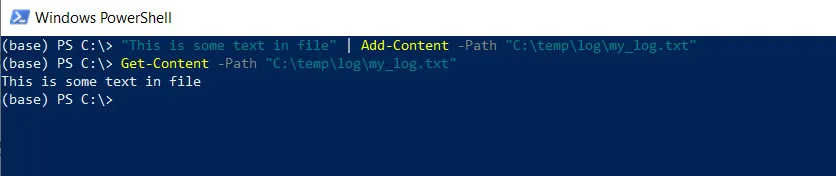
This PowerShell script creates a new file named “my_log.txt” in the “C:\temp\log\” directory and adds the “This is some text in file” to it.
Create a File Using FileStream Class in PowerShell
You can use the .NET System.IO.FileStream
class to create a file in PowerShell
$content = "This is some text in file" $stream = [System.IO.File]::Create("C:\temp\log\my_log.txt") $bytes = [System.Text.Encoding]::UTF8.GetBytes($content) $stream.Write($bytes, 0, $bytes.Length) $stream.Close()
Output:
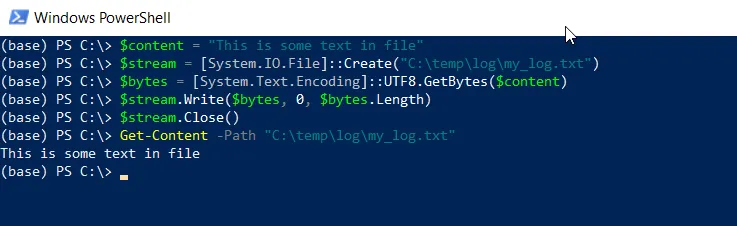
This PowerShell script uses the [System.IO.File]::Create($filePath) to create a file named “my_log.txt“. Next, we convert the $content string into a byte array using the [System.Tex.Encoding]::UTF8.GetBytes($content) and store it in the $bytes variable.
Finally, we call the Write() method on the $stream object, passing it the $byte array, offset 0, and the length of the byte array to create a file and write content to it.
Conclusion
I hope the above article on creating a file in PowerShell using various methods is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.