You can use multiple ways to get the first character of a string in PowerShell.
The following methods show how you can do it with syntax.
Method 1: Using index
$myString = "ActiveDirectoryTools"
$firstChar = $myString[0]
Write-Output "First Character is: $firstChar"
This example will output the first character of a string.
Method 2: Using the Substring method
$myString = "ActiveDirectoryTools"
$firstChar = $myString.Substring(0,1)
Write-Output "First Character is: $firstChar"
This example will output the first character of a string using the Substring() method of a string.
The following examples show how you can use these methods.
Get the First Character of the String Using the Index in PowerShell
You can index in PowerShell to get the first character of a string.
# define a string $myString = "ActiveDirectoryTools" # use indexing $firstChar = $myString[0] # Write the output to the console Write-Output "First Character is: $firstChar"
Output:
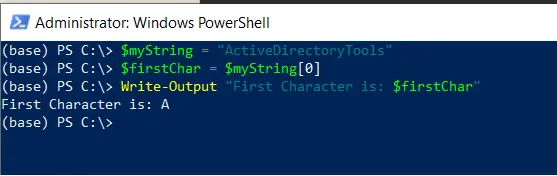
In this PowerShell script, the $myString variable stores the string data “ActiveDirectoryTools“. We then use the index over the string variable like $myString[0] to get the first character of a string.
Finally, the first character of a string is output to the console using the Write-Output cmdlet.
Get the First Character of a String Using the Substring method
You can use the Substring() method of a string to get the first character of a string.
# define a string $myString = "ActiveDirectoryTools" # use substring() method $firstChar = $myString.Substring(0,1) # Write the output to the console Write-Output "First Character is: $firstChar"
Output:
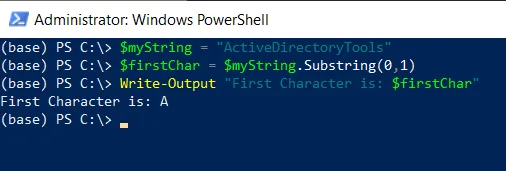
In this script, the $myString variable stores the string data “ActiveDirectoryTools“. We then use the Substring() method over the $myString variable to get the first character of a string.
Finally, the first character of a string is output to the console using the Write-Output cmdlet.
Conclusion
I hope the above article on getting the first character of a string in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.