You can use multiple ways to get the first item in an array using PowerShell.
The following methods show how you can do it with syntax.
Method 1: Using index
$languages = @("PowerShell","Python","Java","C#")
$firstItem = $languages[0]
Write-Output "First item in the array is: $firstItem"
This example will output the first item of an array.
Method 2: Using the Select-Object cmdlet
$languages = @("PowerShell","Python","Java","C#")
$firstItem = $languages | Select-Object -First 1
Write-Output "First item in the array is: $firstItem"
This example will output the first item in an array using the Select-Object cmdlet.
The following examples show how you can use these methods.
Get the First Item in Array Using the Index in PowerShell
You can index in PowerShell to get the first element in an array.
# define an array $languages = @("PowerShell","Python","Java","C#") # use index to get first element of an array $firstItem = $languages[0] # Write the output to the console Write-Output "First item in the array is: $firstItem"
Output:
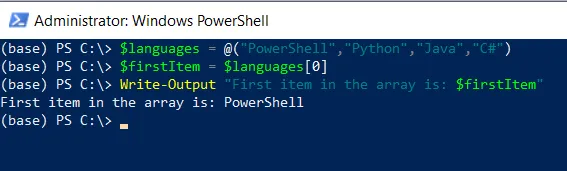
In this PowerShell script, the $languages variable is an array that stores the programming languages. We then use the index over the array variable like $languages[0] to get the first element of an array.
Finally, the first item in an array is output to the console using the Write-Output cmdlet.
Get the First Item in an Array Using the Select-Object Cmdlet
You can use the Select-Object() method with its -First 1 property to get the first item in an array.
# define an array $languages = @("PowerShell","Python","Java","C#") # use Select-Object cmdlet to get first element of array $firstItem = $languages | Select-Object -First 1 # Write the output to the console Write-Output "First item in the array is: $firstItem"
Output:
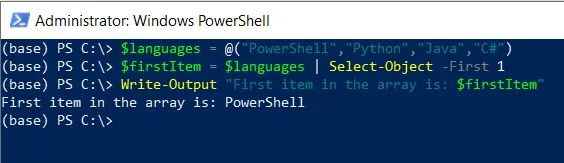
In this script, the $languages variable is an array that stores the programming languages. We then use the Select-Object cmdlet with the -First 1 parameter to get the first item in an array.
Finally, the first item in an array is output to the console using the Write-Output cmdlet.
Conclusion
I hope the above article on getting the first item in an array in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.