PowerShell offers multiple ways to get the month name (eg.. April).
The following methods show how you can do it.
Method 1: Get the month name using GetMonthName() method
# Get the current date
$date = Get-Date
# Get the month number
$monthNumber = $date.Month
# Get the culture
$culture = Get-Culture
# Get the current month name
$monthName = $culture.DateTimeFormat.GetMonthName($monthNumber)
Write-Output "Current Month is: $monthName"
This example will return the current month’s name in PowerShell.
Method 2: Get the month name using AbbrivatedMonthName()
# Get the current date
$date = Get-Date
# Get the month number
$monthNumber = $date.Month
# Get the culture
$culture = Get-Culture
# Get the abbreviated current month name
$abbreviatedMonthName = $culture.DateTimeFormat.GetAbbreviatedMonthName($monthNumber)
Write-Output "Abbreviated current month is: $abbreviatedMonthName"
This example will output the abbreviated current month name.
The following examples show how you can use these methods.
Get Month Name Using GetMonthName() method
The following PowerShell script shows how you can get the month name using the GetMonthName() method.
# Get the current date $date = Get-Date # Get the month number $monthNumber = $date.Month # Get the culture $culture = Get-Culture # Get the current month name $monthName = $culture.DateTimeFormat.GetMonthName($monthNumber) Write-Output "Current Month is: $monthName"
Output:
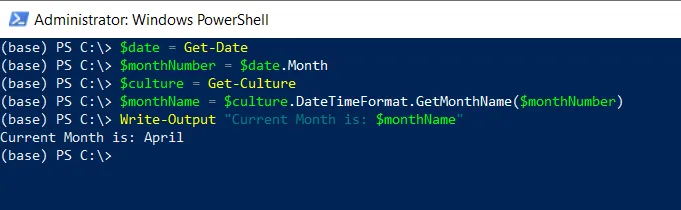
In this PowerShell script, the $date variable stores the current date. $monthNumber variable contains the month number.
We use the Get-Culture cmdlet to get the culture and use the DateTimeFormat property to call the GetMonthName() method.
The $monthName variable stores the month name, If the current month name is April, the output will be “Current Month is: April“.
Get Month Name Using GetAbbreviatedMonthName() method
You can also get the abbreviated month name using the GetAbbreviatedMonthName() method.
The following example shows how you can do it with syntax.
# Get the current date $date = Get-Date # Get the month number $monthNumber = $date.Month # Get the culture $culture = Get-Culture # Get the abbreviated current month name $abbreviatedMonthName = $culture.DateTimeFormat.GetAbbreviatedMonthName($monthNumber) Write-Output "Abbreviated current month is: $abbreviatedMonthName"
Output:
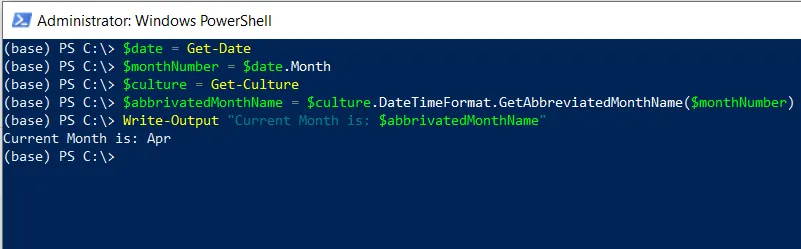
In this PowerShell script, the $date variable stores the current date. $monthNumber variable contains the month number.
We use the Get-Culture cmdlet to get the culture and use the DateTimeFormat property to call the GetAbbreviatedMonthName() method to get the abbreviated month name.
After running the script, this will output the abbreviated current month name. For example, if the current month is April, the output will be “Abbreviated current month is: Apr“.
Conclusion
I hope the above article on getting the month’s name in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.