To remove blank lines from a CSV file using PowerShell, you can use the Import-CSV cmdlet to read the CSV file and then Where-Object cmdlet to filter out blank lines.
The following method shows how you can do it with syntax.
Method 1: Remove blank lines from CSV file
Import-Csv -Path $csvFilePath | Where-Object { $_.PSObject.Properties.Value -ne '' }
This example will import csv file and remove empty lines from a CSV file.
The following example shows how you can use this method.
Remove Empty Lines from CSV File in PowerShell
Suppose that we have empData.csv file that contains multiple empty lines in it. Refer the screenshot where we have used the Get-Content to read the file content.
The following PowerShell syntax shows how you can do it.
# specify the csv file path $csvFilePath = "C:\temp\log\empData.csv" # Read the file content Get-Content -Path $csvFilePath # import the csv file and remove blank lines $csvData = Import-Csv -Path $csvFilePath | Where-Object { $_.PSObject.Properties.Value -ne '' } # export data to csv file $csvData | Export-Csv -Path "C:\temp\log\empData_updated.csv" -NoTypeInformation
Output:
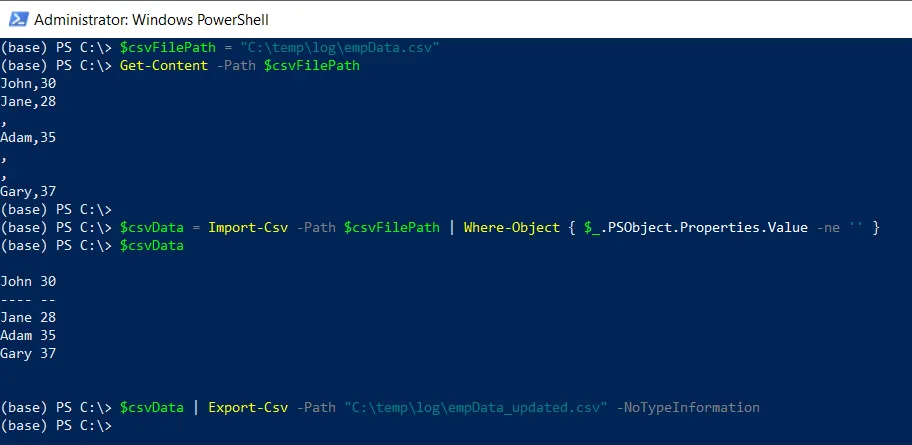
In this script, we define a variable $csvFilePath that stores the path to the csv file.
We then use the Get-Content cmdlet to read the csv file content.
We then use the Import-Csv cmdlet to import the csv file specified by the file path $csvFilePath and reads the csv file content. It pipes the csv file content to the Where-Object cmdlet.
The Where-Object cmdlet checks if the line contains whitespace. If the line is empty, it will not be included in the $csvData variable.
Finally, we use the Export-Csv cmdlet to save the updated CSV content to a new file.
After running the script, it will reads the CSV file and filters out any lines with empty values.
Conclusion
I hope the above article on getting CSV file content and removing blank lines from a CSV file in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.