To remove spaces from a string in PowerShell, you can use the Replace() method or -replace operator.
The following methods show how you can do it with syntax.
Method 1: Using the Replace() method
"This text contains whitespaces in it".Replace(" ", "")
This example will replace all occurrences of spaces in the string with an empty string.
Method 2: Using the -replace operator
"This text contains whitespaces in it" -replace " ", ""
This example will replace whitespace in the string with an empty string.
The following examples show how you can use these methods.
How to Remove Spaces from String Using Replace() Method
Suppose that we have a variable $stringWithWhiteSpaces that contains the string having multiple white spaces in it.
$stringWithWhiteSpaces = “This is a string with spaces”
The following script shows how you can do it.
# define a variable $stringWithWhiteSpaces = "This is a string with spaces" # use the Replace() method to remove spaces $stringWithoutWhiteSpaces = $stringWithWhiteSpaces.Replace(" ", "") Write-Output $stringWithoutWhiteSpaces
Output:
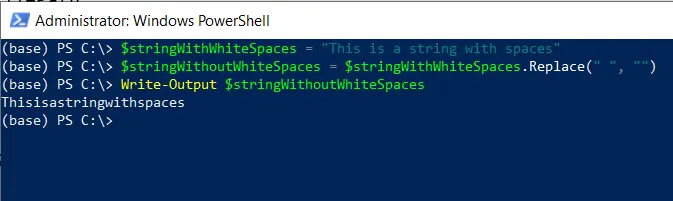
In this script, we define a variable $stringWithWhiteSpaces that contains a string data with whitespace in it.
To remove spaces from a string, we have used the Replace() method that replaces all occurrences of spaces with an empty string and store the result in the $stringWithoutWhiteSpaces variable.
Finally, we use the Write-Output cmdlet to display the string data that contains no spaces in it.
After running the script, it removes all spaces with an empty string and display string on the console.
How to Remove Spaces from String Using -replace Operator
Suppose that we have a variable $stringWithWhiteSpaces that contains the string having multiple white spaces in it.
$stringWithWhiteSpaces = “This is a string with spaces”
The following script shows how you can do it.
# define a variable $stringWithWhiteSpaces = "This is a string with spaces" # use the -replace operator to remove spaces $stringWithoutWhiteSpaces = $stringWithWhiteSpaces -replace " ", "" Write-Output $stringWithoutWhiteSpaces
Output:
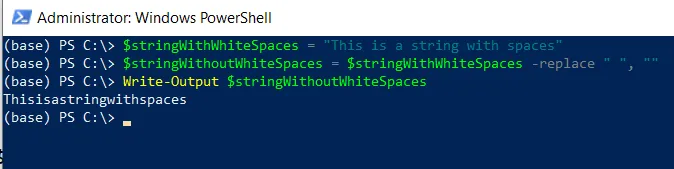
In this script, we define a variable $stringWithWhiteSpaces that contains a string data with whitespace in it.
To remove spaces from a string, we have used the -replace operator that replaces all occurrences of spaces with an empty string and store the result in the $stringWithoutWhiteSpaces variable.
Finally, we use the Write-Output cmdlet to display the string data that contains no spaces in it.
After running the script, it removes all spaces with an empty string and display string on the console.
Conclusion
I hope the above article on removing spaces from a string in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.