To get only the property values without any additional object information, you can use the -ExpandProperty parameter with the Select-Object cmdlet.
The following methods show how you retrieve only the property values in the PowerShell.
Method 1: Using the Select-Object -ExpandProperty
Get-Process | Select-Object -ExpandProperty Name -First 5
This example will return only the property values for the first 5 processes in Windows.
Method 2: Using the ForEach-Object
# Create an object with properties
$object = [PSCustomObject]@{
Property1 = "C#"
Property2 = "PowerShell"
}
# Retrieve only the value of Property1
$propertyValue = $object | ForEach-Object { $_.Property1 }
$propertyValue
This example will return the value of the specified property without any additional object information.
The following examples show how you can use these methods.
Use the Select-Object -ExpandProperty to Get Only Property Value
You can use the -ExpandProperty parameter of the Select-Object command to retrieve the values of the specified property.
# Get the first 5 process and their values only $processList = Get-Process | Select-Object -ExpandProperty Name -First 5 # Output the process values Write-Output $processList
Output:
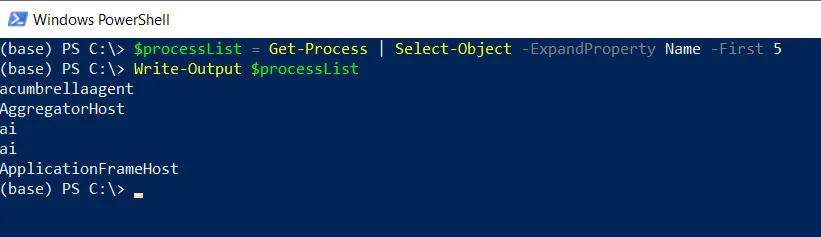
In this example, we define the $processList variable. The Get-Process cmdlet retrieves the list of all processes and pipes them to the Select-Object cmdlet. It then uses the -ExpandProperty
parameter to get only values of the process name and stores them in the $processList
.
Finally, we use the Write-Output cmdlet to output the values of the specified property stored in the $processList
.
The output shows it lists the property values without a header.
Using the ForEach-Object to Get Only Property Value
You can use the ForEach-Object loop to iterate through the selected objects and extract the specified property values.
# Create an object with properties $object = [PSCustomObject]@{ Property1 = "PowerShell" Property2 = "C#" } # Retrieve only the value of Property1 $propertyValue = $object | ForEach-Object { $_.Property1 } Write-Host $propertyValue
Output:
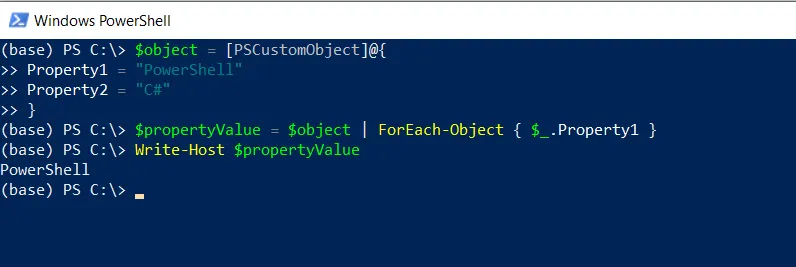
In this example, we created an object with properties using [PSCustomObject]
.
We then pipe the object to the ForEach-Object to retrieve the value of the property Property1
and assign it to the $propertyValue
variable.
Finally, we use the Write-Host cmdlet to output the property value.
Conclusion
I hope the above article on retrieving only property values using the Select-Object cmdlet -ExpandProperty parameter in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.