You can use the Select-Object cmdlet in PowerShell to select multiple properties from an object.
The following methods show how you can do it with syntax.
Method 1: Selecting multiple properties from the Command
$processList = Get-Process | Select-Object Name, Id, CPU -First 5
$processList
This example will extract multiple properties of the Get-Process cmdlet using the Select-Object command.
Method 2: Selecting multiple properties from the custom object
$object = [PSCustomObject]@{
Property1 = "PowerShell"
Property2 = "C#"
}
$selectedProperties = $object | Select-Object Property1, Property2
$selectedProperties
This example will select the multiple properties from the custom object.
The following examples show how you can use these methods.
Selecting Multiple Properties from the Command
You can use the Select-Object cmdlet to extract multiple properties from the command output.
# Get the first 5 processes and select multiple properties $processList = Get-Process | Select-Object Name, Id, CPU -First 5 # Output the processes with selected properties Write-Output $processList
Output:
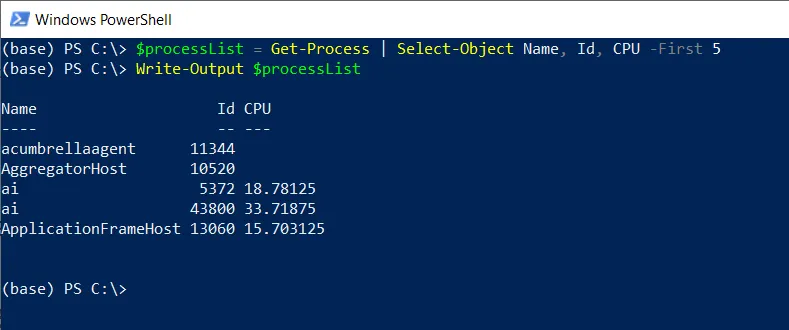
In this example, the Get-Process cmdlet gets processes and pipes them to the Select-Object cmdlet to select multiple properties such as Name
, Id
, and CPU
. The -First 5
parameter selects the first 5 processes and stores the result in the $processList
variable.
We then use the Write-Output cmdlet to output the processes with selected properties.
Selecting Multiple Properties from the Custom Object
You can extract specific information from complex objects, such as custom objects. Here’s how you can do it.
# Create a custom object $object = [PSCustomObject]@{ Property1 = "PowerShell" Property2 = "C#" } # Select multiple properties $selectedProperties = $object | Select-Object Property1, Property2 # Output the selected properties of an object Write-Output $selectedProperties
Output:
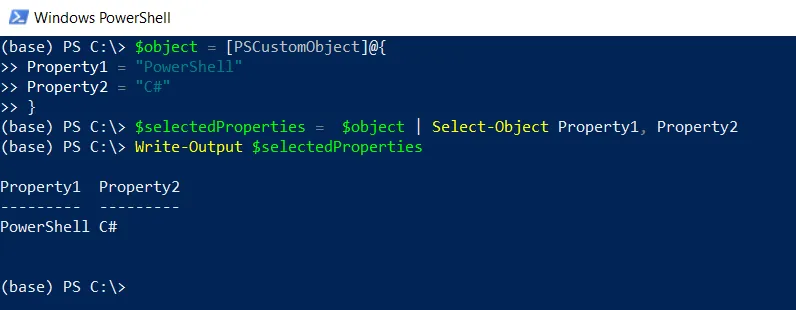
In this example, we create a custom object using the [PSCustomObject]
that includes multiple properties such as Property1 and Property2.
We then pipe the $object
to the Select-Object cmdlet to select the multiple properties and store the result in the $selectedProperties
variable.
Finally, we use the Write-Output cmdlet to output the multiple properties of the custom object.
Conclusion
I hope the above article on selecting multiple properties using the Select-Object cmdlet in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.