You can use multiple ways to slice array in PowerShell.
The following methods show how you can do it with syntax.
Method 1: Slice from the beginning to a specific index
$languages = @("PowerShell","Python","Java","C#")
$slicedArray = $languages[0..2]
Write-Output "Sliced array is: $slicedArray"
This example will slice the array from the beginning to the specific index.
Method 2: Slice from the specific index to the end
$languages = @("PowerShell","Python","Java","C#")
$slicedArray = $languages[1..($languages.Length -1)]
Write-Output "Sliced array is: $slicedArray"
This example will slice the array from a specific index to the end and output the sliced array.
Method 3: Slice with a specific step
$languages = @("PowerShell","Python","Java","C#","Go")
$step = 2
$slicedArray = @()
for($i = 0; $i -lt $languages.Length; $i += $step)
{
$slicedArray += $languages[$i]
}
Write-Output $slicedArray
This example will output the sliced array with a specific step (every nth element).
The following examples show how you can use these methods.
Slice Array from Beginning to Specific Index in PowerShell
You can use array indexing to slice arrays in PowerShell. Array indexing allows you to specify a range of elements to extract from the array.
# Define an array $languages = @("PowerShell","Python","Java","C#") # Use indexing to slice array $slicedArray = $languages[0..2] # Output the sliced array Write-Output "Sliced array is: $slicedArray"
Output:
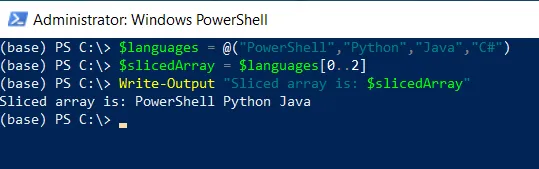
In this script, the $languages variable is an array that contains the programming languages. We then use the array indexing to specify the beginning index and specific index to extract a range of elements from the array.
Finally, we use the Write-Output cmdlet to print the sliced array $slicedArray.
Slice Array from a Specific Index to the End in PowerShell
You can slice an array from a specific index to the end in PowerShell by using the array indexing.
# define an array $languages = @("PowerShell","Python","Java","C#") # slice the array from specific index to the end $slicedArray = $languages[1..($languages.Length -1)] # output the sliced array Write-Output "Sliced array is: $slicedArray"
Output:
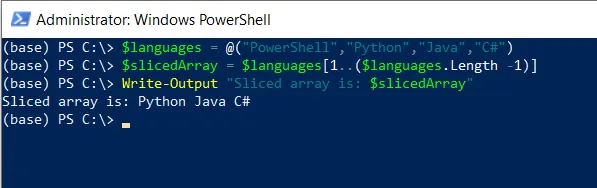
In this script, the $languages variable is an array that contains the programming languages. We then use the array indexing to specify the specific index and end index to extract a range of elements from the array.
Finally, we use the Write-Output cmdlet to print the sliced array $slicedArray.
Slice Array With a Specific Step (Every nth Element) in PowerShell
To slice an array with a specific step (every nth element), you can use a for loop to iterate over the array elements and step to extract a range of items from an array.
# define an array $languages = @("PowerShell","Python","Java","C#","Go") # define step size $step = 2 #initialize an empty array to store the sliced elements $slicedArray = @() # Loop through the array with the specified step size for($i = 0; $i -lt $languages.Length; $i += $step) { $slicedArray += $languages[$i] } # write the output sliced array Write-Output $slicedArray
Output:
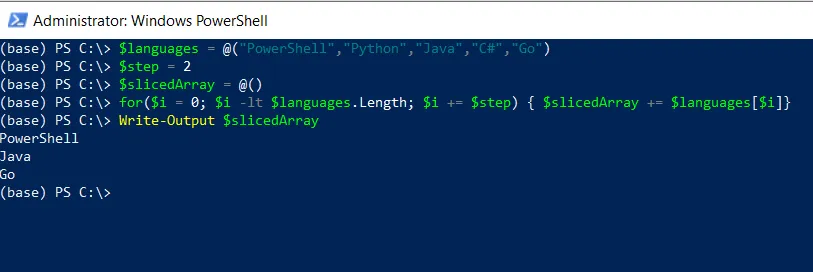
In this script, the $languages variable is an array that contains the programming languages. The $step variable stores the step size.
We then initialize the $slicedArray to store the sliced elements of an array. We use the for loop to iterate through the array elements with the specified step size and extract the range of items from the array.
Finally, we use the Write-Output cmdlet to print the sliced array.
Conclusion
I hope the above article on slice array in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.