In PowerShell, you can split a string into variables using the Split() method or the -split operator, then assign the resulting array elements to the individual variables.
The following methods show how you can do it with syntax.
Method 1: Using the Split() method
$empDetails = "Gary,Smith,37"
$parts = $empDetails.Split(",")
$firstName, $lastName , $age = $parts
This example will split a string and assign the array elements to multiple variables.
Method 2: Using the -split operator
$empDetails = "Gary,Smith,37"
$parts = $empDetails -split ','
$firstName, $lastName , $age = $parts
This example will use the -split operator to split a string and assign the resulting array elements to multiple variables.
The following examples show how you can use these methods.
How to Split String into Variables Using Split() method
In PowerShell, you can call the Split() method on the string to split it into multiple variables.
# specify the string variable $empDetails = "Gary,Smith,37" # use the Split() method to split a string into parts $parts = $empDetails.Split(",") # assign the parts (elements of array) to individual variable $firstName, $lastName , $age = $parts # output the variable Write-Output "First Name: $firstName" Write-Output "Last Name: $lastName" Write-Output "Age: $age"
Output:
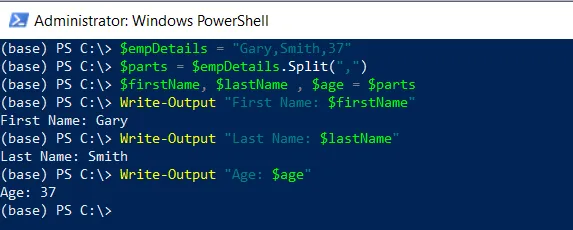
In this script, we define a variable $empDetails the store the string value.
We then use the Split() method with comma (“,“) as the separator to split a string into array elements and assign it to the $parts variable and assign them to the separate variables.
Finally, the Write-Output cmdlet prints the individual part value to the console.
How to Split String into Multiple Variables Using -split Operator
Another way to split a string into multiple variables is to use the -split operator.
# define a string $empDetails = "Gary,Smith,37" # use the -split to split a string into multiple parts $parts = $empDetails -split ',' # assign array of elements to individual variable $firstName, $lastName , $age = $parts # print the variable value Write-Output "First Name: $firstName" Write-Output "Last Name: $lastName" Write-Output "Age: $age"
Output:
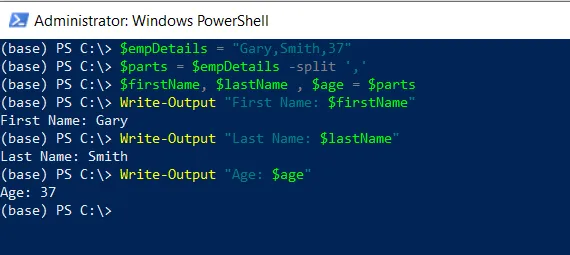
In this script, we define a variable $empDetails that stores the string data.
We then use the -split operator to split the $empDetails string into multiple parts ( array of elements) and assign them to the $parts variable.
The $parts variable values are assigned to the individual variables such as $firstName, $lastName, and $age.
Finally, the Write-Output cmdlet prints the variable value onto the console.
Conclusion
I hope the above article on splitting a string into multiple variables in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.