You can use replace double quotes in a string in PowerShell using the -replace operator or the Replace() method.
Method 1: Using the -replace Operator to replace double quotes in a string
$new_string = $original_string -replace '"', $replacement_string
Method 2: Using the Replace() method to replace double quotes in a string
$new_string = $original_string.Replace('"', $replacement_string
Both of these method replaces the double quotes in a string using the $replacement_string
and store the result in $new_string
.
The following example demonstrates how to use these methods to replace double quotes in a string.
Using the -replace Operator to Replace Double Quotes in a String
The -replace operator in PowerShell replaces the double quotes with the provided $replacement_string value in a string.
# Define the original string $original_string = "Adam replied, ""Hello there!""" # Define the replacement string $replacement_string = '' # Replace double quotes with the replacement string $new_string = $original_string -replace '"', $replacement_string # Output the modified string Write-Output $new_string
In this example, $original_string
variable stores the string that contains the double quotes (“). We have defined the $replacement_string
as a blank. Using the -replace
operator, it replaces the double quotes in a string with the $replacement_string
.
Finally, the modified string is output using the Write-Output
cmdlet.
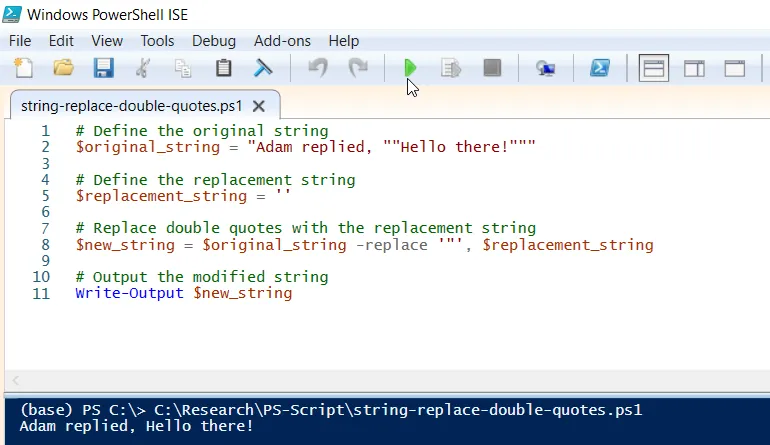
You can also replace double quotes with single quotes in a string in PowerShell.
# Define the original string $original_string = "Adam replied, ""Hello there!""" # Replace double quotes with the replacement string $new_string = $original_string -replace '"', '''' # Output the modified string Write-Output $new_string
The output of the above PowerShell script is given below:
Adam replied, 'Hello there!'
Using the Replace() Method to Replace Double Quotes in a String
The Replace() method is the simplest method to replace double quotes in a string. It matches the double quotes in a string and replaces it with the provided replacement string.
The following PowerShell script demonstrates the practical use of the Replace() method.
# Define the original string $original_string = "Adam replied, ""Hello there!""" # Define the replacement string $replacement_string = '' # Replace double quotes with the replacement string $new_string = $original_string.Replace('"', $replacement_string) # Output the modified string Write-Output $new_string
The output of the above script is given below.
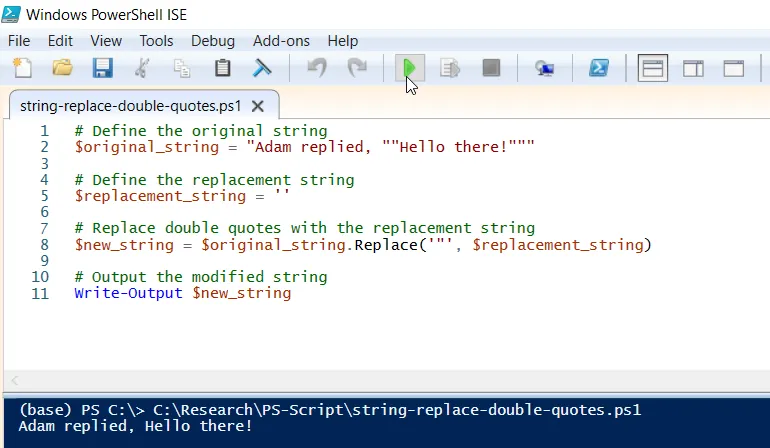
Conclusion
I hope the above article on how to use -replace operator and Replace() method to replace double quotes in a string in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.