PowerShell offers multiple methods to replace special characters in a string in PowerShell. Two common methods are using the -replace
operator with regular expressions or the Replace()
method for string replacements.
Method 1: Using -replace operator to replace special characters
$new_string = $original_string -replace '$#', '')
Method 2: Using Replace() method to remove special characters
$new_string = $original_string.Replace('$#",'')
Both methods look for the special characters in a string and replace them with a replacement string.
The following examples demonstrate the practical application of each method for special character replacements in a string.
Using -replace Operator to Replace Special Characters
The -replace
operator uses regular expressions to match and replace special characters in a string in PowerShell.
The following PowerShell script replaces the special characters in a string.
# Define the original string $original_string = "This !@#string$%^contains&*() special characters" # Define the pattern for special characters $pattern = '[!@#$%^&*()]' # Define the replacement string $replacement = '' # Replace special characters with the replacement string $new_string = $original_string -replace $pattern, $replacement # Output the modified string Write-Output $new_string
In this example, $original_string variable contains the string with special characters. The $pattern variable defines the pattern for special characters.
$replacement
variable defines the replacement string. The -replace
operator matches the given $pattern
in the $original_string
and replaces them with the $replacement
string, in this case, space and store the results in $new_string
.
Finally, the modified string is output using the Write-Output cmdlet in PowerShell.
The following output shows how to use the -replace operator.
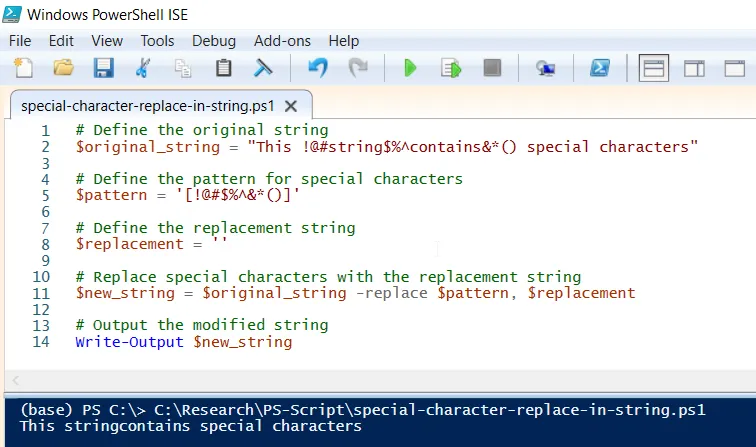
$new_string
stores the result of replacing the special characters in the original string with the replacement string.
Using the Replace() Method to Replace Special Characters
The Replace()
method is a string method that replaces the special characters without using regular expressions.
The following PowerShell script demonstrates how to use the Replace()
method.
$original_string = "The string $!)#()contains special characters" $new_string = $original_string.Replace('$!)#()', ' ') Write-Output $new_string
This example replaces the special characters in a string with the space.
The output of the script is given below.
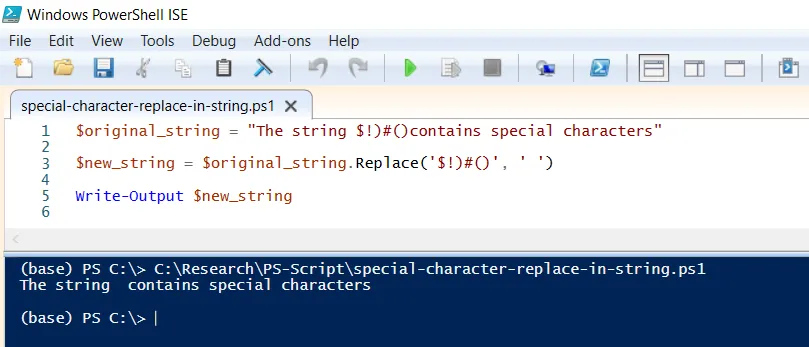
The Replace()
method in PowerShell takes special characters and replacement string as input parameters. It matches the pattern and replaces the special characters with the replacement string, and stores the result in $new_string
.
Conclusion
I hope the above article on how to use the -replace operator and Replace() method in PowerShell to replace special characters in a string.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.