PowerShell offers multiple ways to replace multiple strings in a file in PowerShell. Two common methods are using the -replace
operator and Replace()
method for multiple string replacements in a file.
Method 1: Replace Multiple Strings in a File Using -replace Operator
$original_string = Get-Content -Path C:\temp\file1.txt -Raw
$new_string = $original_string -replace "old_text", "new_text" -replace "old_text1", "new_text1"
Method 2: Replace Multiple Strings in a File Using Replace() Method
$original_string = Get-Content -Path C:\temp\file1.txt -Raw
$new_string = $original_string.Replace("old_text","new_text").Replace("old_text1","new_text1")
Both methods replace the multiple strings with the new string in a file named file.txt. It replaces old_txt with new_txt and old_txt1 with new_txt1.
The following examples demonstrate the practical application of each method to replace multiple strings in a file.
Replace Multiple Strings in a File Using -replace Operator
We can chain -replace
operations together to replace multiple strings in a file in PowerShell.
The file.txt contains the below information.
Adam has scored 90% in Statistics in his final semister of graduation. Adam is now pursuing master degree in Statistics.
We want to perform the following replacements in a file:
- Replace “Adam” with “Gary“.
- Replace “Statistics” with “Computer Science“.
The following example replaces the multiple strings in a file.
$original_string = Get-Content -Path C:\temp\file1.txt -Raw $new_string = $original_string -replace "Adam", "Gary" -replace "Statistics", "Computer Science" Write-Output $new_string
The output of this example replaces every occurrence of the old string with new strings in a file.
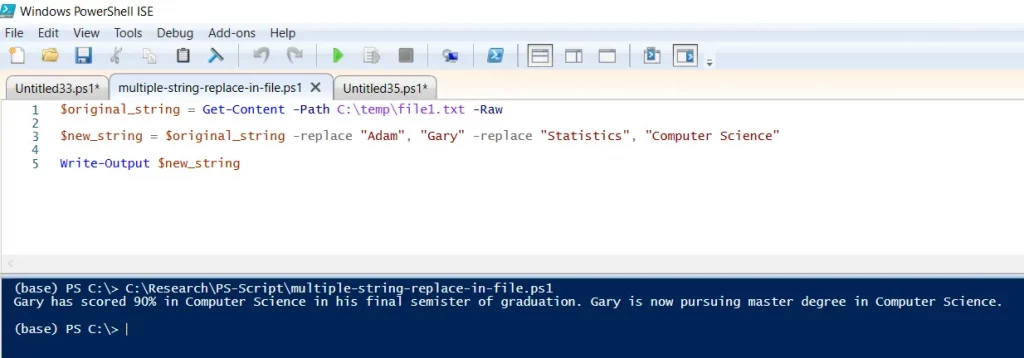
The Get-Content
cmdlet is used to read the content of the file and store it in the $original_string
variable. It uses the -Raw parameter to read the file content as a single string object.
We have chain -replace
operations to replace multiple strings and store the result in the $new_string
variable. Finally, we have used the Write-Output
cmdlet to output the modified string.
Replace Multiple Strings in a File Using Replace() Method
We can use the Replace() method to replace multiple strings in a file using PowerShell. It replaces every occurrence of old strings with new strings.
The following PowerShell script replaces the “Adam” with “Gary” and “Statistics” with “Computer Science” in a file.
$original_string = Get-Content -Path C:\temp\file1.txt -Raw $new_string = $original_string.Replace("Adam", "Gary").Replace("Statistics", "Computer Science") Write-Output $new_string
The output of this example replaces every occurrence of the old string with new strings in a file.
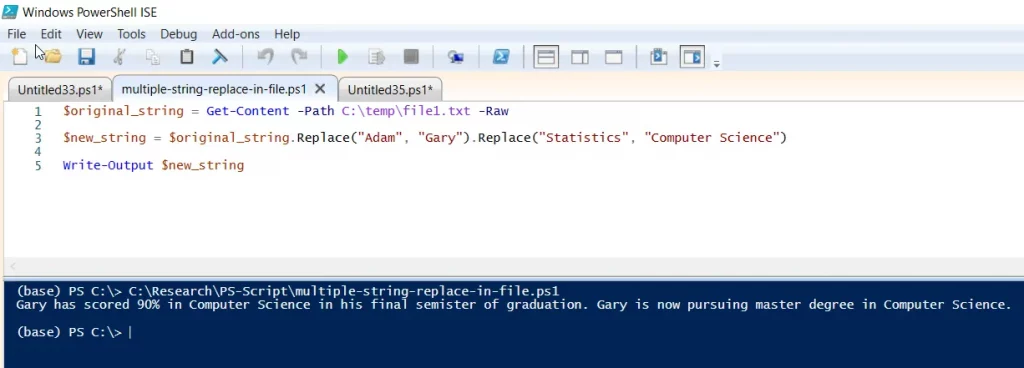
The Get-Content
cmdlet is used to read the content of the file and store it in the $original_string
variable. It uses the -Raw
parameter to read the file content as a single string object.
We have chain Replace()
operations to replace multiple strings and store the result in the $new_string
variable. Finally, we have used the Write-Output
cmdlet to output the modified string.
Conclusion
I hope the above article on how to replace multiple strings in a file in PowerShell using the -replace operator and Replace() method is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.