To check if a string exists in the list of strings in PowerShell, you can use the -in operator or the Contains() method or -contains operator.
The following methods show how you can do it with syntax.
Method 1: Using the -in operator
$languages = "Java","Python","C#","Go"
$searchString = "C#"
$searchString -in $languages
This example will check if C# exists in the list $languages. It will return either True or False.
Method 2: Using the Contains() method
$languages = "Java","Python","C#","Go"
$searchString = "C#"
$languages.Contains($searchString)
This example uses the Contains() method to check the $searchString exists in the list variable $languages and returns either True or False.
Method 3: Using the -contains operator
$languages = "Java","Python","C#","Go"
$searchString = "C#"
$languages -contains $searchString
This example uses the -contains operator to check if the $searchString (C#) exists in the list $languages and returns either True or False.
The following examples show how you can use these methods to check if a string exists in the list of strings in PowerShell.
Check If String Exists in List Using the -in Operator
You can use the -in operator within an if statement to check if a string exists in the list of strings and return either True or False.
# define list $languages = "Java","Python","C#","Go" # define target string $searchString = "C#" # check if string exists in the list if($searchString -in $languages) { Write-Output "The string $searchString is in the list" } else{ Write-Output "The string $searchString is not in the list" }
Output:
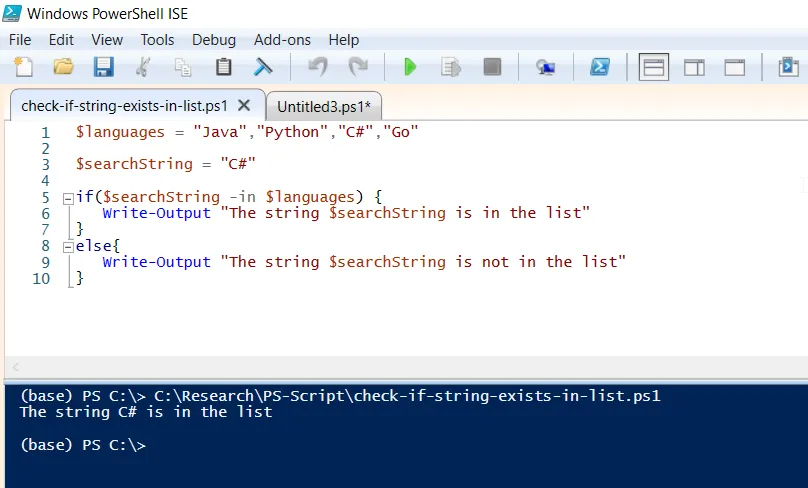
In this example, we define a variable named $languages which contains the list of strings and $searchString with a value of “C#“.
We then use the -in operator in the if statement to check if $searchString (C#) exists in the list named $languages and returns either True or False value.
Finally, after running the PowerShell script, it outputs “The string C# is in the list“.
Check If String Exists in List Using the Contains() Method in PowerShell
You can use the Contains() method directly on the list of strings to check if a string exists in the list of strings and return either True or False.
# define the list $languages = "Java","Python","C#","Go" # define the target string $searchString = "PowerShell" # check if string exists in the list if($languages.Contains($searchString)) { Write-Output "The string $searchString is in the list" } else{ Write-Output "The string $searchString is not in the list" }
Output:
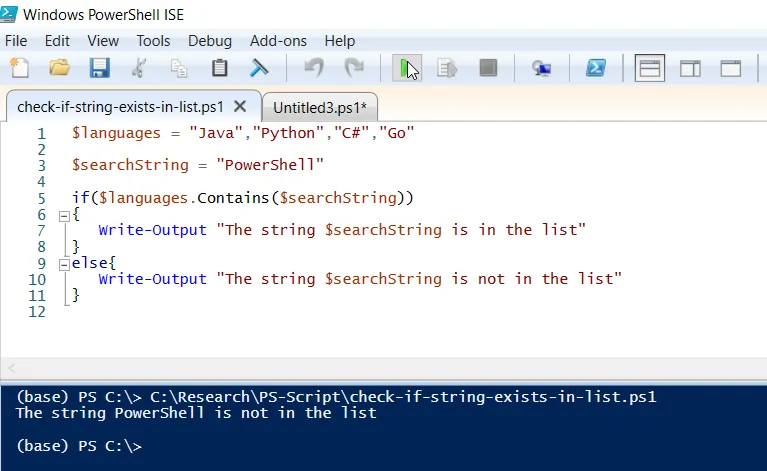
In this example, we define a variable named $languages which contains the list of strings and $searchString with a value of “PowerShell“.
We then use the Contains() method on the list of strings to check if $searchString (PowerShell) exists in the list named $languages and returns either a True or False value.
Finally, after running the PowerShell script, it outputs “The string PowerShell is not in the list“.
Check If String Exists in List Using the -contains Operator in PowerShell
Another way to check if a string exists in the list of strings in PowerShell is to use the -contains operator.
# Define list $languages = "Java","Python","C#","Go" # define the target string $searchString = "Go" # check if string exists in the list if($languages -contains $searchString)) { Write-Output "The string $searchString is in the list" } else{ Write-Output "The string $searchString is not in the list" }
Output:
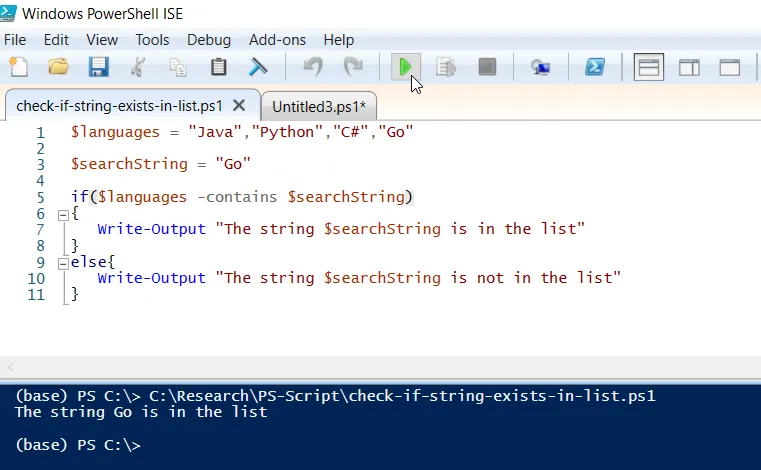
In this example, we define a variable named $languages which contains the list of strings and $searchString with a value of “Go“.
We then use the -contains operator in the if statement to check if $searchString (Go) exists in the list named $languages and returns either True or False value.
Finally, after running the PowerShell script, it outputs “The string Go is in the list“.
Conclusion
I hope the above article on checking if a string exists in the list of strings using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.