You can find the input value or element in an array in PowerShell by using the Contains() method or -contains operator.
The following methods show how you can do it with syntax.
Method 1: Using the Contains() method
if($array.Contains("4")){
Write-Output "4 is in the array"
}
else {
Write-Output "4 is not in the array"
}
This example checks if element 4 in the array $array and then outputs the result.
Method 2: Using the -contains operator
if($array -contains 3){
Write-Output "3 is in the array"
}
else {
Write-Output "3 is not in the array"
}
This example uses the -contains operator to check if element 3 in the array $array and then outputs the result.
The following examples show how to check the input value in the array in PowerShell using these methods.
Using the Contains() Method
The following PowerShell script shows how you can use the Contains() method.
# define array $languages = @("Python","C#","Java","Go") # Check if input value in the array if($languages.Contains("C#")) { Write-Output " C# is in the array" } else{ Write-Output "C# is not in the array" }
Output:
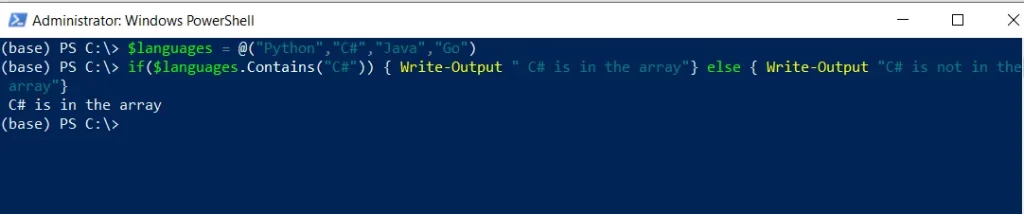
In this example, we define the $languages variable that contains the array elements.
We then use the Contains() method over the $languages array to check if the input value C# in the array and output the result.
In our example, the C# language is in the $languages array, hence it will output the result as “C# is in the array“.
Using the -contains Operator
Another way to check if an element is in an array using PowerShell is to use the -contains operator.
Here’s the PowerShell script.
# define array $languages = @("Python","C#","Java","Go") # Check if input value in the array if($languages -contains "C#") { Write-Output " C# is in the array" } else{ Write-Output "C# is not in the array" }
Output:

In this example, we define the $languages variable that contains the array elements.
We then use the -contains operator to check if the input value C# in the array and output the result.
In our example, the C# language is in the $languages array, hence it will output the result as “C# is in the array“.
Conclusion
I hope the above article on checking if the input value in an array using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.