In PowerShell, you can extract the substring before a specific character using the SubString() method or the -split operator.
The following methods show how you can do it with syntax.
Method 1: Using the Substring Method
$string = "Hello, World!"
$index = $string.IndexOf(',')
$substringBefore = $string.SubString(0,$index)
Write-Output $substringBefore
This example will extract the substring “Hello” from the $string before a specific character comma (,).
Method 2: Using the -split operator
$string = "Hello, World!"
$substringBefore = $string -split ',' | Select -Object -First 1
Write-Output $substringBefore
This example uses the -split operator to split the $string by a specific character comma (,) and extracts the substring “Hello” from the $string before a specific character comma (,).
The following examples show how to use these methods to extract substring before character in PowerShell.
Extract SubString Before Character Using the SubString() Method
To extract a substring before a specific character in PowerShell, you can use a combination of the IndexOf() and SubString() methods.
The IndexOf() method is used to find the position of the character, and the SubString() method is used to extract the substring based on that position.
# Define a string $string = "ActiveDirectoryTools.Net" $index = $string.IndexOf('.') $substringBefore = $string.SubString(0,$index) Write-Output $substringBefore
Output:
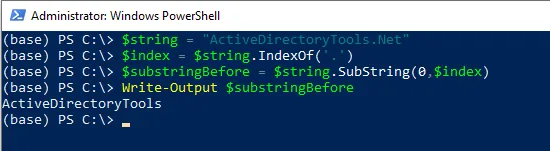
In this example, we define a variable $string that contains the string data “ActiveDirectoryTools.Net“.
We then use the IndexOf() method over the $string to find the position of the character (.) and store it in the $index variable.
Using the $index position, we used the SubString() method to extract the substring before character (.) and store the result in the $substringBefore variable.
Finally, after running the PowerShell script, it outputs the substring before a character, in this case, “ActiveDirectoryTools“.
Extract SubString Before Character Using the split Operator
Another way to extract a substring before a character in PowerShell is to use the -split operator.
# define a string $string = "PowerShell-Active-Directory-Tools" # Split the string at the hyphen (-) and take the first part $substringBefore = $string -split '-' | Select-Object -First 1 # output the result Write-Output $substringBefore
Output:
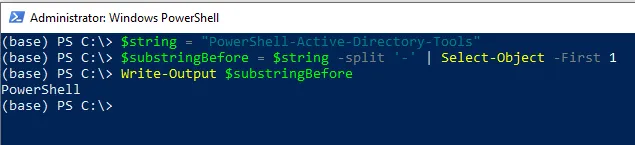
In this example, we define a variable $string that contains the string data “PowerShell-Active-Directory-Tools“.
We then use the -split operator to split the $string at the hyphen (-) and the Select-Object cmdlet to select the first part and store the result in the $substringBefore variable.
Finally, after running the PowerShell, it extracts the substring before a character (-) and prints “PowerShell” on the console.
Conclusion
I hope the above article on extracting the substring before a specific character in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.