There are several ways to check if a file is empty in PowerShell.
The following methods show how to do it with syntax.
Method 1: Check the file size
# specify the path to the file
$filePath = "C:\temp\log\my_log.txt"
if(Get-Item $filePath).Length -eq 0){
Write-Host "File is empty."
} else {
Write-Host "File is not empty."
}
This example checks if the file is empty or not using the Length property of the file object.
Method 2: Check the number of lines
# specify the path to the file
$filePath = "C:\temp\log\my_log.txt"
# get the line count
$lineCount = (Get-Content $filePath | Measure-Object -Line).Lines
if($lineCount -eq 0){
Write-Host "File is empty."
} else {
Write-Host "File is not empty."
}
This example checks if the file is empty or not using the number of lines in a file.
Method 3: Using IsNullOrWhiteSpeace
# specify the path to the file
$filePath = "C:\temp\log\my_log.txt"
if([string]::IsNullOrWhiteSpace((Get-Content -Path $filePath))){
Write-Host "File is empty."
} else {
Write-Host "File is not empty."
}
The following examples show how to use these methods.
Check If a File is Empty Using the File size
You can check if a file is empty in PowerShell by checking the file size.
The following script shows how you can check it.
# specify the path to the file $filePath = "C:\temp\log\my_log.txt" if((Get-Item $filePath).Length -eq 0){ Write-Host "File is empty." } else { Write-Host "File is not empty." }
Output:

In the above PowerShell script, the Get-Item cmdlet uses the -Path parameter to specify the $filePath and retrieve the file object. It uses the file object Length property to check if the file size is equal to 0.
If the file size is equal to 0, it will display “File is empty.” otherwise “File is not empty.” using the Write-Host cmdlet in PowerShell.
In the above output, it displays the “File is empty.” message on the console.
Check If a File is Empty Using the Number of Lines in the File
You can check if a file is empty by counting the number of lines in the file. If the number of lines in the file is 0, it means the file is empty otherwise the file is not empty.
# specify the path to the file $filePath = "C:\temp\log\my_log.txt" # get the line count $lineCount = (Get-Content $filePath | Measure-Object -Line).Lines if($lineCount -eq 0){ Write-Host "File is empty." } else { Write-Host "File is not empty." }
Output:
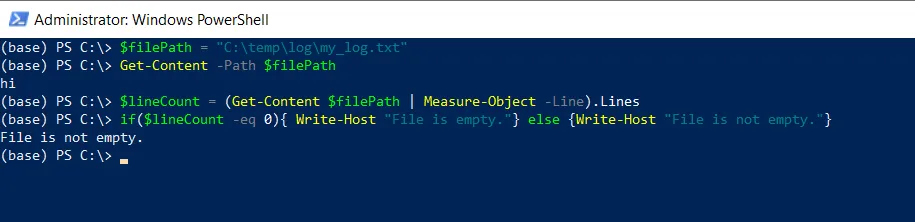
In this example, the Get-Content cmdlet reads the file content specified by the $filePath variable and pipes the output to the Measure-Object. The Measure-Object cmdlet uses the -Line switch to count the number of lines in the file.
If the number of lines ($lineCount) is equal to 0, the “File is empty.” otherwise “File is not empty.“
Check if a File is Empty Using Null or White Space
Another way to check if a file is empty is by checking the file content for any characters other than null or whitespace.
# specify the path to the file $filePath = "C:\temp\log\my_log.txt" if([string]::IsNullOrWhiteSpace((Get-Content -Path $filePath))){ Write-Host "File is empty." } else { Write-Host "File is not empty." }
Output:
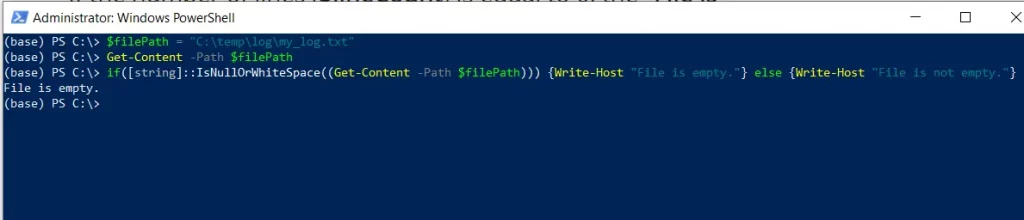
In this script, the $filePath variable contains the full path to the file you want to check for empty. The Get-Content cmdlet with the $filePath variable reads the content of the file.
The [string]::IsNullOrWhiteSpace() method is used to check if the content contains only null or whitespace characters.
If the condition is true, the file is considered to be empty, and “File is empty.” is printed to the console.
If the condition is false, the file contains non-whitespace characters, and “File is not empty.” is printed to the console.
Conclusion
I hope the above article to check if a file is empty in PowerShell using the various methods is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.