In PowerShell, you can use the Where-Object cmdlet with not null condition to filter out objects having a null value in the specific property.
The following method shows how you can do it with syntax.
Method 1: Filter out objects having null values using Where-Object
$employees = @(
[PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" },
[PSCustomObject]@{ Name = "Gary"; Age = $null; Department = "Sales" },
[PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" }
)
$result = $employees | Where-Object { $_.Age -ne $null}
$result
This example will filter out employees having a $null
value in the Age and return employees with a non-null age.
The following example shows how you can use this method.
Filtering Empty Value or Object having Null Value Using Where-Object
Suppose we have an array of custom objects representing employees with properties Name, Age, and Department.
We want to filter out only those objects where the Age
property is not null
.
# Define an array of custom objects representing employees $employees = @( [PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" }, [PSCustomObject]@{ Name = "Gary"; Age = $null; Department = "Sales" }, [PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" } ) # Use Where-Object to filter employees where Age is not null $result = $employees | Where-Object { $_.Age -ne $null} # Output the filtered result $result
Output:
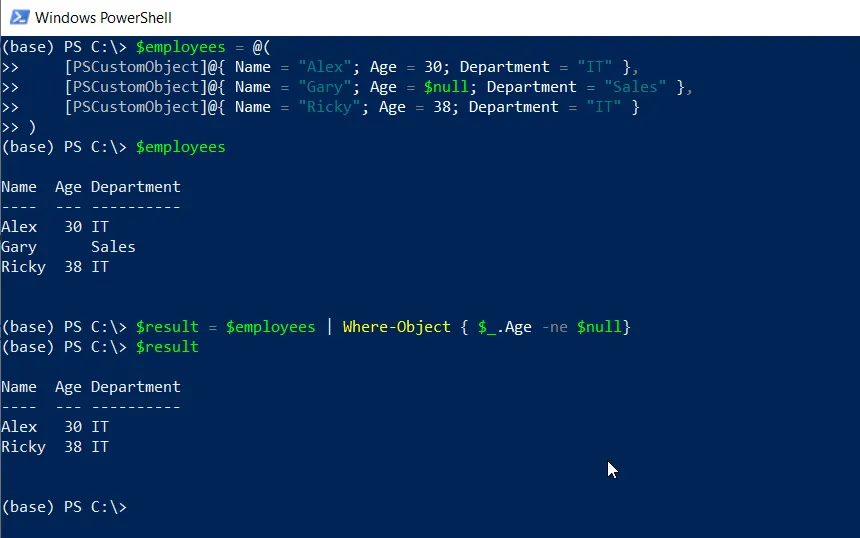
PowerShell Where-Object with not null
In this example, the $employees
variable is an array of custom objects with properties Name
, Age
, and Department
.
We then pipe the $employees
to the Where-Object to filter the array to include objects where the Age property is not equal to $null
and store the result in the $result
.
Finally, we print the filtered results on the terminal.
Conclusion
I hope the above article on filtering empty or objects having null values using the Where-Object cmdlet with no null condition in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.