In PowerShell, you can use the Where-Object cmdlet with a logical operator -and
to combine multiple conditions.
The following methods show how you can do it with syntax.
Method 1: Filtering with Where-Object and -And
$employees = @(
[PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" },
[PSCustomObject]@{ Name = "Gary"; Age = 35; Department = "Sales" },
[PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" }
)
$result = $employees | Where-Object { $_.Age -gt 35 -and $_.Department -eq "IT"}
$result
This example will return the employees having age greater than “35” and who belong to the “IT” department.
Method 2: Filtering with Where-Object and -and for Strings
$languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go")
$result = $languages | Where-Object { $_ -like "C*" -and $_.length -eq 2}
$result
This example will return languages “C#”.
The following examples show how you can use these methods.
Filtering with Where-Object and -And
Suppose you have an array of custom objects representing employees, and you want to filter for employees having age more than 35 years and belong to the IT department.
# Define an array of custom objects $employees = @( [PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" }, [PSCustomObject]@{ Name = "Gary"; Age = 35; Department = "Sales" }, [PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" } ) # Use Where-Object with the -and operator to filter the array $result = $employees | Where-Object { $_.Age -gt 35 -and $_.Department -eq "IT"} # Output the filtered result $result
Output:
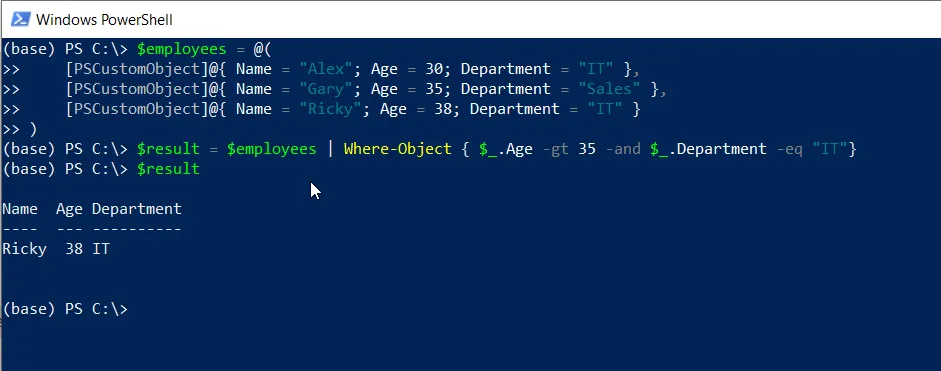
In this example, we define $employees
array of custom objects with properties Name
, Age
, and Department
.
We then pipe the $employees
into the Where-Object
cmdlet to filter the array to include objects where the Age
property is greater than 35 and the Department
property is IT and stores the result in $result
.
Finally, we print the $result
on the terminal that displays the filtered result.
Filtering with Where-Object and -and for Strings
Suppose you have an array of strings representing programming languages and want to filter for strings starting with “C” and having a length equal to 2.
# define an array of strings $languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go") # Use the Where-Object with the -and operator to filter strings $result = $languages | Where-Object { $_ -like "C*" -and $_.length -eq 2} # Output the filtered result $result
Output:
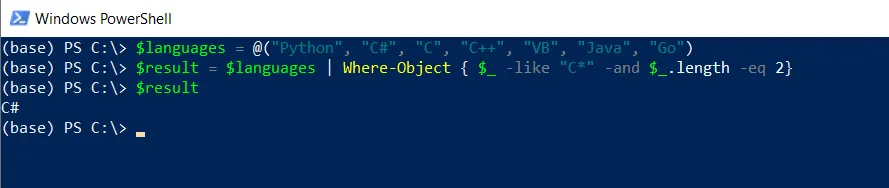
In this example, we define $languages
array that represents programming languages. We then pipe the $languages
to the Where-Object cmdlet to filter for strings.
The Where-Object command uses the -and
operator to specify multiple conditions, including programming languages containing “C*” and length should be equal to 2 and store the result in $result
.
Finally, we print the output on the terminal.
Conclusion
I hope the above article on filtering objects with the Where-Object cmdlet in PowerShell with the -and operator is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.