You can use the Where-Object cmdlet in PowerShell to find an item in a list.
The following method shows how you can do it with syntax.
Method 1: Find an item in the list
$languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go")
$searchItem = "VB"
$foundItem = $languages | Where-Object { $_ -eq $searchItem }
if($foundItem) {
Write-Host "Found '$searchItem' in the list."
} else {
Write-Host "'$searchItem' not found in the list."
}
This example will search for item ‘VB‘ in the list and return if found.
Find an Item in the List in PowerShell
To find an item in the list in PowerShell, you can use the Where-Object cmdlet to filter and compare the item in the list using the -eq
operator.
# Define a list of programming languages $languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go") # Define the search item $searchItem = "VB" # Find specific search item in the list using Where-Object $foundItem = $languages | Where-Object { $_ -eq $searchItem } if($foundItem) { Write-Host "Found '$searchItem' in the list." } else { Write-Host "'$searchItem' not found in the list." }
Output:
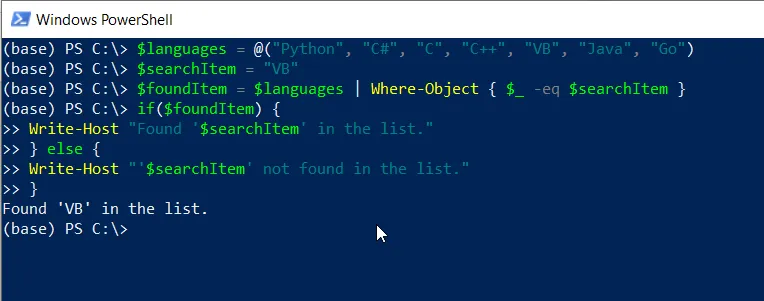
In this example, we define a list $languages
representing programming langauges. We then pipe the $languages
to the Where-Object.
The Where-Object cmdlet filters the array to include only items that match “VB” and store the result in $foundItem
.
Finally, we use the if-else statement to check if the $searchItem
found in the list and print it on the terminal.
Conclusion
I hope the above article on finding item in the list in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.