You can convert string to DateTime object in PowerShell using multiple ways.
The following methods show how you can do it with syntax.
Method 1: Using the Parse() method
$dateString = "2024-04-16"
# Convert the string to a DateTime object
$converted_date = [DateTime]::Parse($dateString)
$converted_date
This example will convert the string to a DateTime object.
Method 2: Using the Get-Date cmdlet with the -Date parameter
$dateString = "2024-04-16"
# Convert the string to a DateTime object
$converted_Date = Get-Date -Date $dateString
$converted_Date
This example will convert the string to a DateTime object by using the Get-Date cmdlet.
The following examples show how you can use these methods.
Convert String to DateTime Using Parse() Method
To convert a string to a DateTime object in PowerShell, you can use the Parse() method of the [DateTime] class.
$dateString = "2024-04-16" # Convert the string to a DateTime object $converted_Date = [DateTime]::Parse($dateString) $converted_Date
Output:
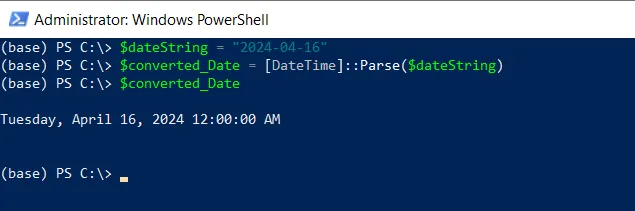
In this script, the $dateString variable stores the datetime in string format. The Parse() method of the [DateTime] class converts the $dateString into a DateTime object and stores it in the $converted_Date variable.
Finally, we output the $converted_Date to the console.
Convert String to DateTime Using Get-Date cmdlet
Another way to convert a string to a DateTime object is to use the Get-Date cmdlet with the -Date parameter.
$dateString = "2024-04-16" # Convert the string to a DateTime object $converted_Date = Get-Date -Date $dateString $converted_Date
Output:
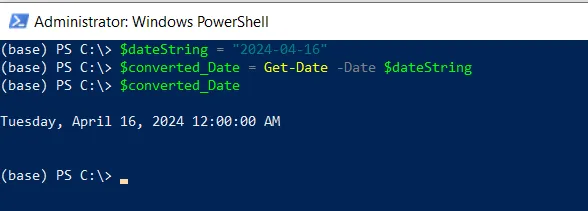
In this script, the Get-Date cmdlet uses the -Date parameter to convert the $dateString to a DateTime object and store it in the $converted_Date variable.
Finally, we output the $converted_Date to the console.
Conclusion
I hope the above article on converting a string to a DateTime object in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.