To find duplicate values in an array in PowerShell, you can use the Group-Object cmdlet and filter out the groups with a count greater than 1.
The following method shows how you can do it.
Method 1: Using the Group-Object cmdlet
$numArray = @(10,2,4,2,90,2,5,76,4)
$duplicates = $numArray | Group-Object | Where-Object { $_.Count -gt 1}
Write-Output "Duplicate values in the arrays are: "
$duplicates | Foreach-Object {
Write-Object $.Name
}
This example will output the duplicate values.
The following example shows how you can do it.
Find Duplicate Values in Array Using PowerShell
You can use the Group-Object cmdlet in PowerShell to group the elements of an array by their values and use Where-Object to filter out duplicate values based on specified criteria.
$numArray = @(10,2,4,2,90,2,5,76,4) $duplicates = $numArray | Group-Object | Where-Object { $_.Count -gt 1} Write-Output "Duplicate values in the arrays are: " $duplicates | Foreach-Object { Write-Output $.Name }
Output:
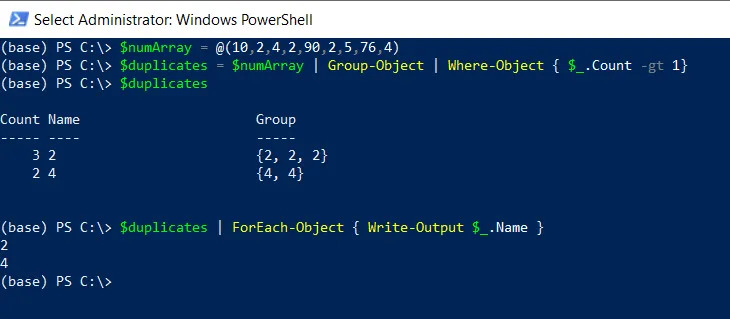
In this script, the $numArray variable stores the array. It then pipes to the Group-Object cmdlet to group the elements of the $numArray by their values.
The Where-Object cmdlet then filters out the groups with a count greater than 1, which represents the duplicate values.
Finally, the Write-Output cmdlet outputs the duplicate values in an array.
Conclusion
I hope the article on finding the duplicate values in an array is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.