To find empty folders in PowerShell, you can use the Get-ChildItem cmdlet to recursively search through the directories and filter the results based on whether the directory contains any child items (files or folders).
The following method shows how you can do it.
Method 1: Find empty folders
# specify the directory path
$directoryPath = "C:\temp\log\"
# Get all directories and filter for empty folders
$emptyFolders = Get-ChildItem -Path $directoryPath -Directory -Recurse | Where-Object {$_.GetFileSystemInfos().Count -eq 0}
(base) PS C:\> $e
Write-Output $emptyFolders
This example will display the empty folder names.
The following example shows how to use this method.
Find Empty Folders in PowerShell
You can use the Get-ChildItem cmdlet in PowerShell to recursively search for a directory using the -Recurse parameter and then filter folders based on whether they contain any files or folders in them using the GetFileSystemInfos() method.
# specify the directory path $directoryPath = "C:\temp\log\" # Get all directories and filter for empty folders $emptyFolders = Get-ChildItem -Path $directoryPath -Directory -Recurse | Where-Object {$_.GetFileSystemInfos().Count -eq 0} (base) PS C:\> $e Write-Output $emptyFolders
Output:
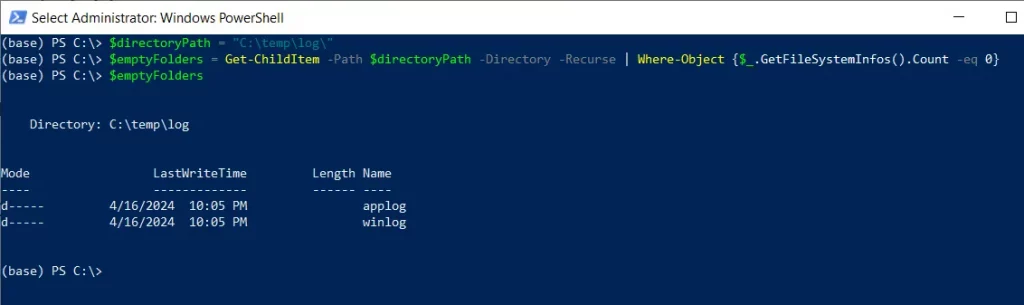
In this script, the $directoryPath variable stores the base directory path in which you want to find empty folders.
The Get-ChildItem command uses the -Path and -Directory parameters to retrieve the subdirectories within the specified $directoryPath recursively.
It then pipes the output to the Where-Object command to filter the directories to include only those that contain no child items (files or subfolders).
Finally, the Write-Output cmdlet in PowerShell outputs the empty folder names.
After running the script, it displays two empty folders “applog” and “winlog“.
Conclusion
I hope the above article on getting the empty folders in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.