To exclude certain files or directories using the Get-ChildItem in PowerShell, you can use the -Exclude parameter.
The following methods show how you can do it with syntax.
Method 1: Exclude specific files using Get-ChildItem
Get-ChildItem -Exclude "syslog.txt","temp.txt"
This example will retrieve all other files in the current directory excluding syslog.txt and temp.txt files.
Method 2: Exclude specific directories using Get-ChildItem
Get-ChildItem -Directory -Exclude "Syslog"
This example will retrieve all other directories in the current directory excluding the syslog folder.
Method 3: Exclude specific files with a pattern using Get-ChildItem
Get-ChildItem -Exclude "*.txt"
This example will exclude files with an extension .txt while retrieving the files in the current directory.
Method 4: Exclude directories with a pattern using Get-ChildItem
Get-ChildItem -Directory -Exclude "Sys*"
This example will exclude the folder that starts with “Sys*“.
The following examples show how you can use these methods.
How to Exclude Specific Files Using the Get-ChildItem
Use the Get-ChildItem cmdlet with the -Exclude parameter to specify the list of files that you want to exclude.
# specify the directory path $folderPath = "C:\temp\log" # exclude specific files in the directory $files = Get-ChildItem -Path $folderPath -Exclude "syslog.txt","temp.txt" Write-Output $files
Output:
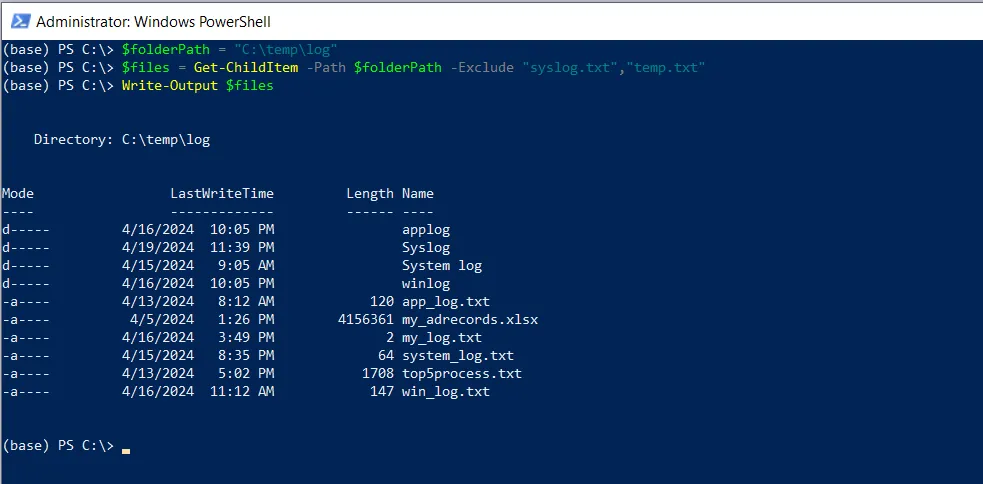
In this script, we define a variable $folderPath that contains the directory path.
We then use the Get-ChildItem cmdlet along with its -Exclude parameter to specify the “syslog.txt” and “temp.txt” files and store all other files in the $files variable.
Finally, we use the Write-Output cmdlet to output the list of files excluding the “syslog.txt” and “temp.txt” files.
How to Exclude Specific Directories Using Get-ChildItem
You can exclude the specific directories using the Get-ChildItem cmdlet along with the -Exclude parameter to specify the directory names you want to exclude from the result.
# specify the directory path $folderPath = "C:\temp\log" # exclude specific files in the directory $folders = Get-ChildItem -Path $folderPath -Directory -Exclude "syslog" Write-Output $folders
Output:
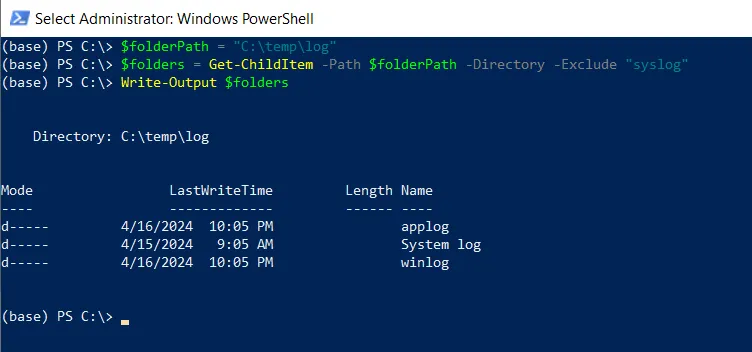
In this script, we define a variable $folderPath to store the directory path.
We then use the Get-ChildItem cmdlet along with its -Directory and -Exclude parameters to specify the directory name “Syslog” to exclude while retrieving all other directories.
Finally, we use the Write-Output cmdlet to output the list of directories excluding the directory “Syslog“.
How to Exclude Specific Files with Extension Using Get-ChildItem
You can use the Get-ChildItem cmdlet along with the -Exclude parameter to specify the file extension that you want to exclude.
# specify the directory path $folderPath = "C:\temp\log" # exclude specific files in the directory $files = Get-ChildItem -Path $folderPath -Exclude "*.txt" Write-Output $files
Output:
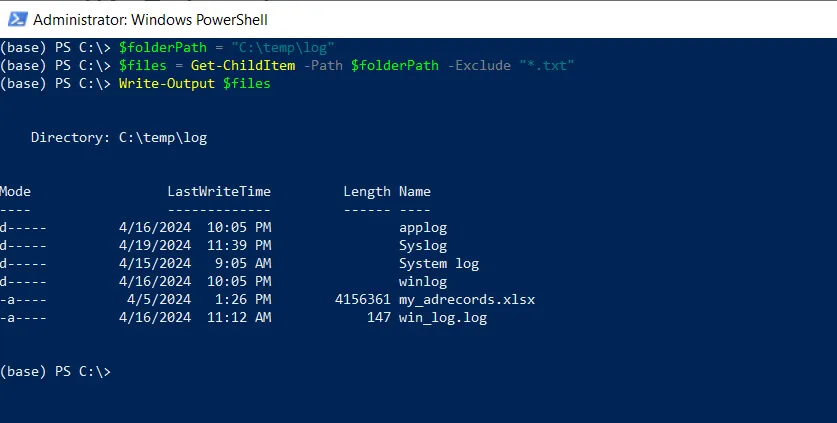
In this script, we define a variable $folderPath that contains the directory path.
We then use the Get-ChildItem cmdlet along with the -Exclude parameter to specify the file extension or pattern you want to exclude in this, “*.txt“
Finally, we use the Write-Output cmdlet to output the list of files excluding the .txt files.
How to Exclude Directories with a Patter Using Get-ChildItem
You can use the Get-ChildItem cmdlet with the -Exclude parameter to specify the pattern to exclude the specific directories.
# specify the directory path $folderPath = "C:\temp\log" # exclude specific files in the directory $folders = Get-ChildItem -Path $folderPath -Directory -Exclude "Sys*" Write-Output $folders
Output:
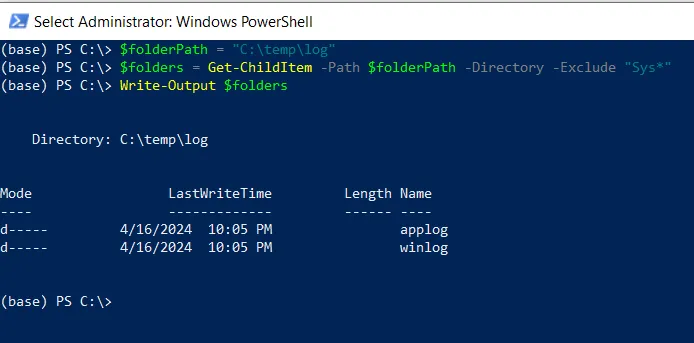
In this script, we define a variable $folderPath that stores the directory path.
We then use the Get-ChildItem cmdlet along with the -Exclude parameter to specify the pattern for directories that you want to exclude from the list.
Finally, we use the Write-Output cmdlet to output the directories excluding the directories that start with “Sys*“.
Conclusion
I hope the above article on how to exclude certain items (files or directories) using the Get-ChildItem cmdlet in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.