To get the list of files by modified date in PowerShell, use the Get-ChildItem cmdlet to retrieve all files and pipe them to the Where-Object to check the LastWriteTime property of the file.
The following methods can be used to list files by modified date.
Method 1: List files modified on a specific date
$date = "2024-04-16"
Get-ChildItem | Where-Object { $_.LastWriteTime.Date -eq $date}
This example will return the list of files modified on a specified date.
Method 2: Find files modified within the last n days
$days = 30
$date = (Get-Date).AddDays(-$days)
Get-ChildItem | Where-Object {$_.LastWriteTime.Date -ge $date}
This example will return the list of files modified in the last 30 days.
Method 3: Find files modified between two dates
$startDate = "04-10-2024"
$endDate = "04-16-2024"
Get-ChildItem | Where-Object {$_.LastWriteTime.Date -ge $startDate -and $_.LastWriteTime.Date -le $endDate}
This example will return the files modified between two dates.
The following examples show how you can use these methods.
How to Get Files Modified on Specific Date Using Get-ChildItem in PowerShell
The following syntax shows how you can do it.
# specify the folder path $folderPath = "C:\temp\log" #specify the date $date = "2024-04-16" # get the list of files modified on the date Get-ChildItem | Where-Object { $_.LastWriteTime.Date -eq $date}
Output:
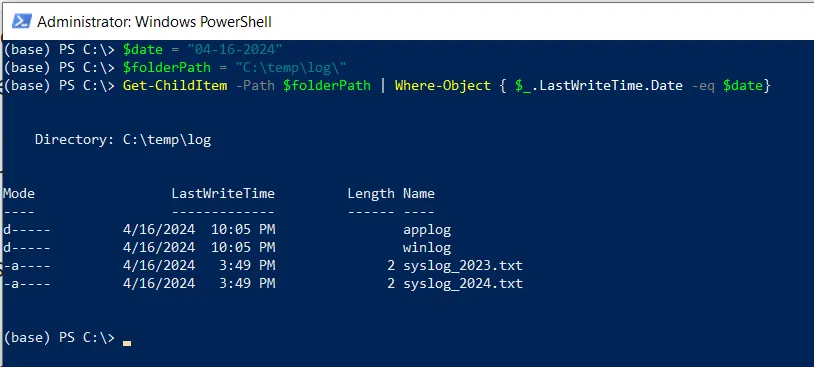
In this script, we define a variable $folderPath that contains the directory path, and $date variable stores the specific date.
We then use the Get-ChildItem cmdlet to retrieve all files from the specified $folderPath and pipe them to the Where-Object cmdlet.
The Where-Object command uses the search criteria to check if the LastWriteTime property to the file is equal to the specific date and returns the list of files.
After running the script, it will display the list of files modified on a specific date.
How to Find Files Modified Within the Last N Days with Get-ChildItem in PowerShell
To find files modified between the last n days, use the Get-ChildItem cmdlet to retrieve all files and then filter them by using the Where-Object cmdlet.
# specify the folder path $folderPath = "C:\temp\log" #specify the days $days = 30 # get the date before last 30 days $date = (Get-Date).AddDays(-$days) # Get files modified within last 30 days Get-ChildItem -Path $folderPath | Where-Object {$_.LastWriteTime.Date -ge $date}
Output:
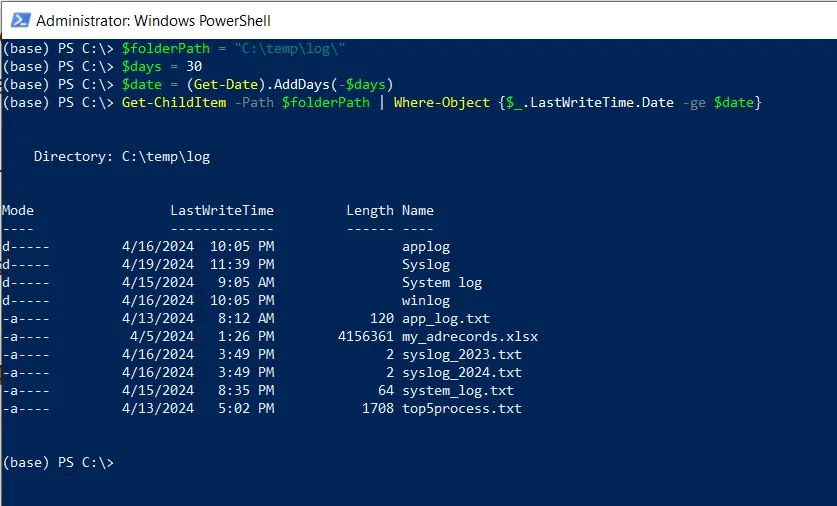
In this script, the Where-Object cmdlet checks if the LastWriteTime property of each file is greater than the specified $date.
After running the script, it lists the files modified in the last 30 days.
How to Find Files Modified Between Two Dates Using Get-ChildItem
To find files modified between two dates in PowerShell, use the Get-ChildItem cmdlet to retrieve files and then the Where-Object command to check the LastWriteTime property of the file with specified two dates.
# specify the folder path $folderPath = "C:\temp\log" #specify two dates $startDate = "04-10-2024" $endDate = "04-16-2024" # get the files between two dates Get-ChildItem -Path $folderPath | Where-Object {$_.LastWriteTime.Date -ge $startDate -and $_.LastWriteTime.Date -le $endDate}
Output:
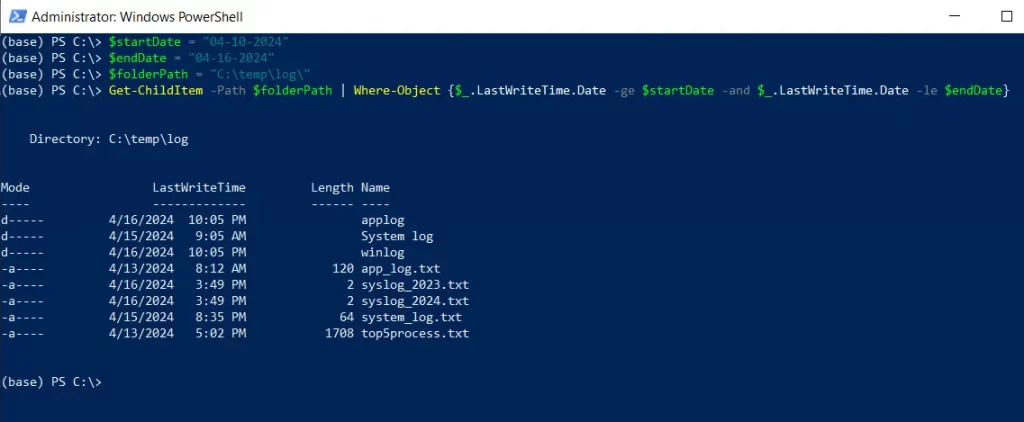
In this script, we define two variables $startDate and $endDate which contain the datetime objects.
We then use the Get-ChildItem cmdlet to retrieve all files from the $folderPath and pipe them to the Where-Object cmdlet.
The Where-Object command uses the search criteria to select the files modified between the two date ranges by checking the LastWriteTime property of the file with the $startDate and $endDate.
After running the script, it will return the list of files modified within two dates in PowerShell.
Conclusion
I hope the above article on getting childitem by modified date using the Get-ChildItem cmdlet in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.