In PowerShell, there are several ways you can get content and skip the first N lines in PowerShell.
The following methods show how you can do it with syntax.
Method 1: Using the Get-Content with -Skip 1 parameter
Get-Content -Path $filePath -Skip 1
This example will read the file content and skip the first 1 line.
Method 2: Using the Get-Content with array indexing
$fileData = Get-Content -Path $filePath
$fileData[1..($fileData.Length)]
This example will read the file content and select all the lines of the file except the first one.
The following examples show how you use these methods.
Get Content and Skip the First Line of a File Using Skip Parameter
You can use the Get-Content cmdlet to read the file content and the Select-Object cmdlet with the -Skip parameter to skip the first line of a file.
# specify the file path $filePath = "C:\temp\log\system_log.txt" # Get content and skip the first line of a file Get-Content -Path $filePath | Select-Object -Skip 1
Output:
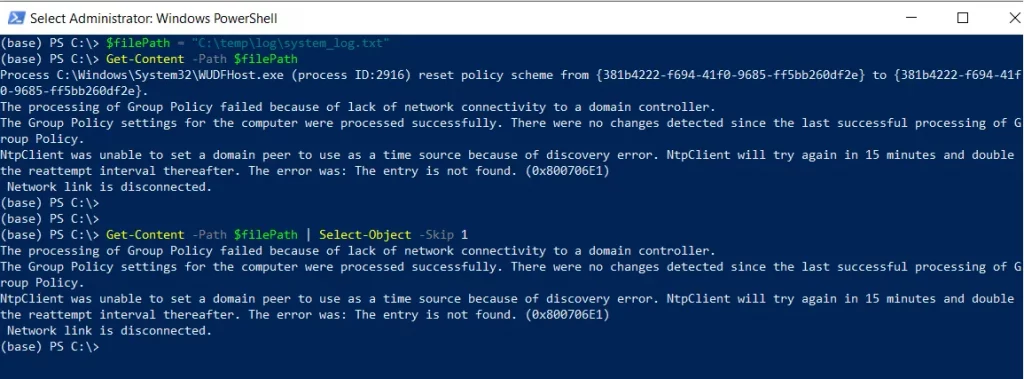
In this script, we define a variable $filePath that stores the path to the file.
We then use the Get-Content cmdlet to get the content and pipe it to the Select-Object cmdlet. The Select-Object command in combination with the -Skip 1 parameter skips the first line of the file.
After running the script, it will display the content of the file starting from the second line.
If you want to skip N lines, let’s say the first 10 lines of a file, you can use the same command with some modification in the -Skip parameter.
# specify the file path $filePath = "C:\temp\log\system_log.txt" # Get content and skip the first line of a file Get-Content -Path $filePath | Select-Object -Skip 10
After running this script, it will display the file content starting from the 11th line.
Get Content and Skip the First Line of a File Using Array Indexing Parameter
Another way to get content and skip the first line of a file is to use array indexing in PowerShell.
# specify the file path $filePath = "C:\temp\log\system_log.txt" # Get content and skip the first line of a file $fileData = Get-Content -Path $filePath $fileData[1..($fileData.Length)]
Output:
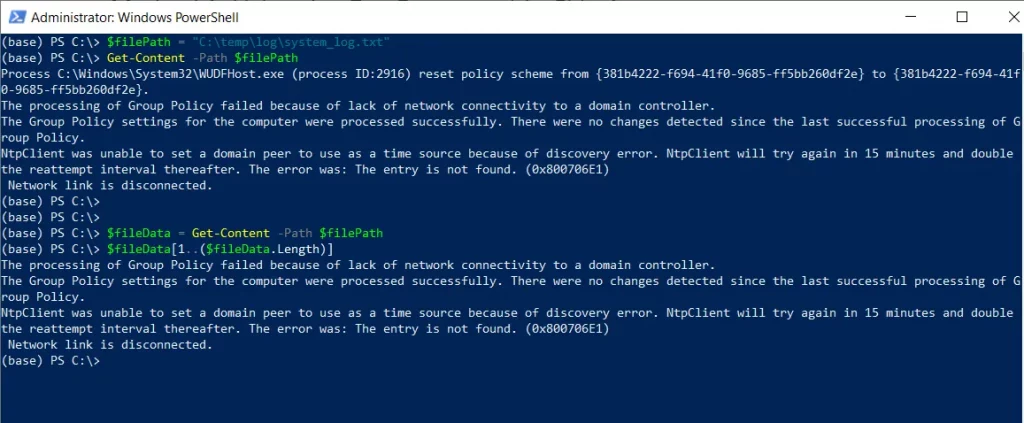
In this script, we define a variable $filePath that stores the path to the file.
We then use the Get-Content cmdlet to get the content of the $filePath and store it in the $fileData variable.
To skip the first line of a file, we used the array indexing. The $fileData[1..($fileData.Length )] selects all the lines of the file except the first one.
After running the script, it will display the file content starting from the second line.
You can skip N lines using the array indexing with some modifications in the array indexing.
# specify the file path $filePath = "C:\temp\log\system_log.txt" # Get content and skip the first line of a file $fileData = Get-Content -Path $filePath $fileData[5..($fileData.Length)]
After running this script, it will skip the first 5 lines of a file.
Conclusion
I hope the above article on getting the content and skipping the first line of a file in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.