To get file properties in PowerShell, you can use Get-Item, Get-ChildItem, or Get-ItemProperty cmdlets to retrieve the file information.
The following methods can be used to get file attributes in PowerShell.
Method 1: Get file properties using the Get-Item cmdlet
# Specify the file path
$filePath = "C:\temp\log\system_log.txt"
# Retrieve the file information
Get-Item -Path $filePath
This example will display the file details such as file name, length, mode, and lastwritetime.
Method 2: Get the file information using Get-ChildItem cmdlet
# Specify the file path
$filePath = "C:\temp\log\system_log.txt"
# Retrieve the file information
Get-ChildItem -Path $filePath
This example will display the file information such as file name, length, mode, and lastwritetime.
Method 3: Get the file details using the Get-ItemProperty cmdlet
# Specify the file path
$filePath = "C:\temp\log\system_log.txt"
# Retrieve the file information
Get-ItemProperty -Path $filePath
This example will display the file metadata such as file name, length, mode, and lastwritetime.
The following examples show how to use these methods.
Get file properties using the Get-Item cmdlet
You can retrieve the file properties using the Get-Item cmdlet in PowerShell. It returns the file name, mode, length, and lastwritetime attributes.
# Specify the file path $filePath = "C:\temp\log\system_log.txt" # Retrieve the file information Get-Item -Path $filePath
Output:
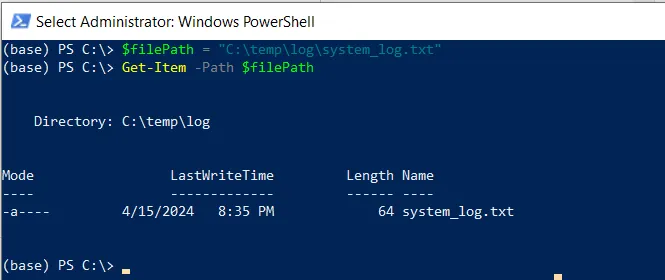
The Get-Item cmdlet uses the -Path parameter to specify the file path, retrieves the file properties, and displays it to the console.
Get the file information using the Get-ChildItem cmdlet
Another way to get the file information is by using the Get-ChildItem cmdlet in PowerShell. It returns the file metadata such as file name, mode, length, and lastwritetime.
# Specify the file path $filePath = "C:\temp\log\system_log.txt" # Retrieve the file information Get-ChildItem -Path $filePath
Output:
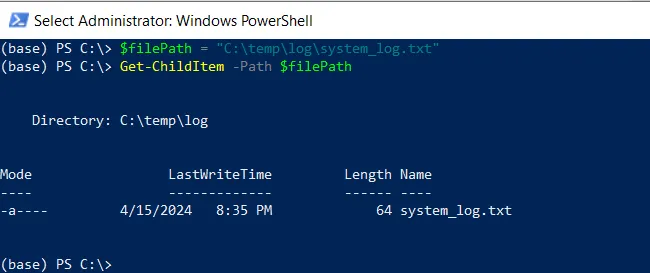
The Get-ChildItem cmdlet in PowerShell retrieves the file object properties and displays them to the console.
Get the file details using the Get-ItemProperty cmdlet
You can get the file details using the Get-ItemProperty cmdlet in PowerShell. It gets the properties of the specified file.
# Specify the file path $filePath = "C:\temp\log\system_log.txt" # Retrieve the file information Get-ItemProperty -Path $filePath
Output:
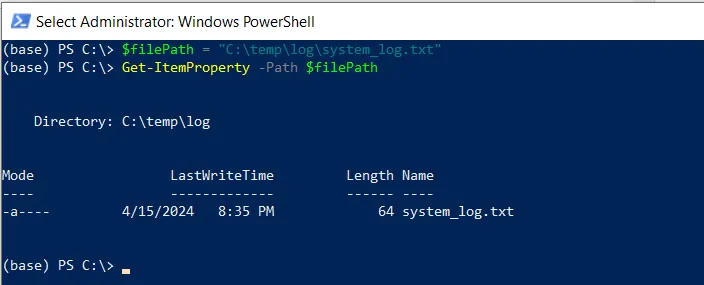
The Get-ItemProperty cmdlet in PowerShell retrieves the properties of the file specified by the $filePath variable and displays them to the console.
Conclusion
I hope the above article on getting file properties in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.