CSV ( Command-Separated Values) files are widely used to store tabular data. CSV files often have headers in their first row, providing the column names that enhance the data clarity. However, there might be instances when you need to work with a CSV file that doesn’t have headers.
In this article, we will discuss how to import csv files without headers in PowerShell using the `Import-Csv
` cmdlet.
Using Import-CSV without Headers
PowerShell `Import-Csv
` command primarily assumes the presence of headers in the CSV file. However, when working with CSV files that don’t have headers, you can follow the below steps to import csv and manage the data effectively.
Specify Headers Manually
Since the CSV file doesn’t have headers, you can provide column names using the -Header parameter when importing the CSV file using the `Import-Csv
` cmdlet.
Import-CSV -Path D:\PS\team_information.csv -Header "Group","Name","Years"
In the above PowerShell script, the `Import-Csv
` command read the csv content and imports the csv specified by the Path
parameter. It uses the -Header
parameter to specify the column names manually.
The output of the above PowerShell script after adding headers to the CSV file is:
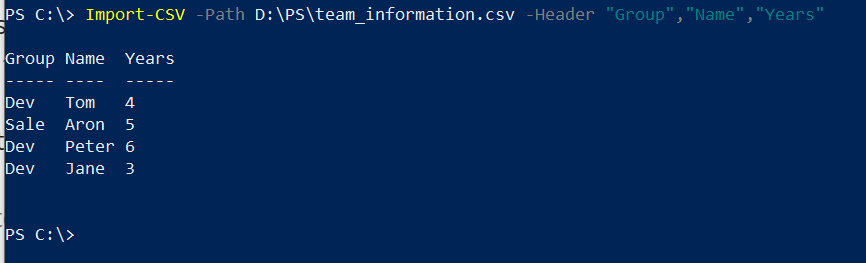
Accessing the Data
Once the CSV data is imported with the provided headers, you can access the contents using the column names you specified.
# import the csv file $csvdata = Import-CSV -Path D:\PS\team_information.csv -Header "Group","Name","Years" # Access the data using the column name foreach ($row in $csvData) { Write-Host "Value in Column1: $($row.Group)" Write-Host "Value in Column2: $($row.Name)" Write-Host "Value in Column3: $($row.Years)" }
The output of the above PowerShell script after accessing the data is:
Value in Column1: Dev
Value in Column2: Tom
Value in Column3: 4
Value in Column1: Sale
Value in Column2: Aron
Value in Column3: 5
Value in Column1: Dev
Value in Column2: Peter
Value in Column3: 6
Value in Column1: Dev
Value in Column2: Jane
Value in Column3: 3
Cool Tip: How to get column names for a CSV file in PowerShell!
Conclusion
I hope the above article on how to import csv without headers in PowerShell is helpful to you.
Importing a csv file without column names can be effectively managed using the `Import-CSV
` cmdlet along with manual column header specification.
Since the CSV file doesn’t have headers, PowerShell considers the first row of the data as the first record. Be careful while handling the data.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.