You can use the Foreach loop to iterate over the objects in an array after importing a CSV file with the Import-CSV cmdlet in PowerShell.
The following method shows how you can do it with syntax.
Method 1: Import CSV with foreach loop
$processArray = Import-CSV -Path $csvFilePath
foreach( $process in $processArray){
$Name = $process.Name
$Id = $process.Id
Write-Output "Name: $Name, Id: $Id"
}
This example will import a CSV file and iterate each row in the CSV file, performing actions with the column values as needed.
The following example shows how you can use this method.
How to Import CSV with Foreach Loop in PowerShell
The following PowerShell script shows how to import csv and iterate using a foreach loop.
# specify the csv file path $csvFilePath = "C:\temp\log\processList.csv" # import csv file $processArray = Import-CSV -Path $csvFilePath # iterate each row using foreach loop foreach( $process in $processArray){ $Name = $process.Name $Id = $process.Id Write-Output "Name: $Name, Id: $Id" }
Output:
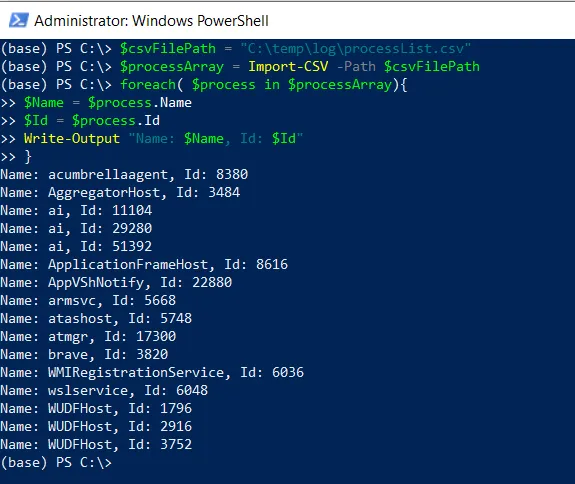
In this script, we define a $csvFilePath variable that stores the csv file path that you want to import.
We then use the Import-CSV cmdlet to import a CSV file and store its content in the $processArray variable.
We used a foreach loop to iterate over each object (row) in the $processArray. You can access the values of specific columns within each row using the column names from the CSV file.
Finally, we output the CSV file content to the console.
Conclusion
I hope the above article on importing a CSV file with a foreach loop in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.