To set or change the owner of a file using PowerShell, you can use the Set-Acl cmdlet.
The following method shows how to do it with syntax.
Method 1: Set the owner of a file using PowerShell
# Specify the path to the file
$filePath = "C:\temp\log\system_log.txt"
# Specify the new owner
$newOwner = "Administrator"
# Get the file's current ACL
$fileAcl = Get-Acl -Path $filePath
# Create a new security identifier (SID) for the new owner
$newOwnerSid = New-Object System.Security.Principal.NTAccount($newOwner)
$newOwnerSid = $newOwnerSid.Translate([System.Security.Principal.SecurityIdentifier])
# Set the owner in the file's ACL
$fileAcl.SetOwner($newOwnerSid)
# Apply the modified ACL to the file
Set-Acl -Path $filePath -AclObject $fileAcl
This example will set the owner of a file in PowerShell. It changes the security descriptor of the file.
The following example shows how to use this method.
How to Set the Owner of a File Using PowerShell
You can set the owner of a file or folder in PowerShell using the Set-Acl cmdlet. It involves changing the security descriptor of a file or descriptor.
PowerShell doesn’t have a built-in cmdlet for setting the owner directly, hence we will be using the .NET classes available in PowerShell to implement it.
# Specify the path to the file $filePath = "C:\temp\log\system_log.txt" # Specify the new owner $newOwner = "Administrator" # Get the file's current ACL $fileAcl = Get-Acl -Path $filePath # Create a new security identifier (SID) for the new owner $newOwnerSid = New-Object System.Security.Principal.NTAccount($newOwner) $newOwnerSid = $newOwnerSid.Translate([System.Security.Principal.SecurityIdentifier]) # Set the owner in the file's ACL $fileAcl.SetOwner($newOwnerSid) # Apply the modified ACL to the file Set-Acl -Path $filePath -AclObject $fileAcl
Output:
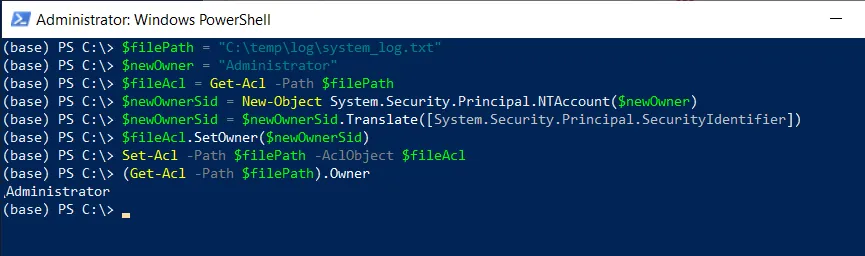
In this PowerShell script, the $filePath variable holds the path to the file you want to set or change owner. The $newOwner variable stores the new owner’s name.
$fileAcl variable stores the file’s current ACL retrieved using the Get-Acl cmdlet, later we created a new security identifier (SID) for the new owner and stored it in the $newOwnerSid variable.
Finally, we set the owner in the file’s ACL using the SetOwner method and applied the modified ACL to the file using the Set-Acl method.
Conclusion
I hope the above article on setting the owner of a file using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.