To set registry value with PowerShell, you can do it using the New-ItemProperty cmdlet or the Set-ItemProperty cmdlet.
You can use the following methods to set the registry value in PowerShell.
Method 1: Set registry value using New-ItemProperty cmdlet in PowerShell
New-ItemProperty -Path $registryPath -Name $propertyName -Value $propertyValue -PropertyType String
In this example, the New-ItemProperty cmdlet sets the registry value of the specified item.
Method 2: Set registry value using Set-ItemProperty cmdlet in PowerShell
Set-ItemProperty -Path $registryPath -Name $propertyName -Value $propertyValue
In this example, the Set-ItemProperty cmdlet creates a new registry entry $propertyName, and assigns a value $propertyValue to it.
The following examples show how to use these methods.
Set Registry Value Using New-ItemProperty Cmdlet in PowerShell
To set the registry value in PowerShell, use the New-ItemProperty cmdlet in PowerShell. It creates a new property for an item and sets its value.
The following example shows how to use it with syntax.
# Specify the path to the registry key $registryPath = "HKLM:\Software\SysApp\" # Specify the name of the registry value $propertyName = "AppKey" # Specify the value data $propertyValue = "0100-0fc00-0bd2-002a" # Sets registry value New-ItemProperty -Path $registryPath -Name $propertyName -Value $propertyValue -PropertyType String
Output:
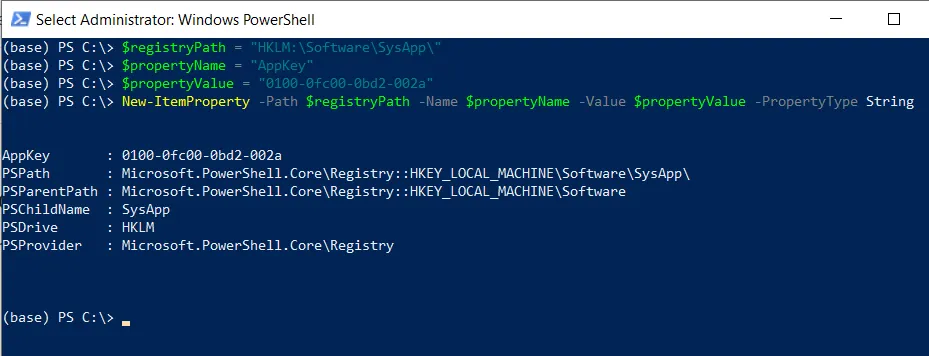
In this example, the $registryPath variable holds the path to the registry key. $propertyName variable holds the name of the registry value you want to set.
The $propertyValue variable stores the data you want to set for the registry value. The -PropertyType parameter in the New-ItemProperty cmdlet specifies the data type of the registry value, in this case, it’s a String
type.
The output shows the New-ItemProperty sets the registry value for the specified item.
Set Registry Value Using Set-ItemProperty Cmdlet in PowerShell
To set registry value in PowerShell, you can use the Set-ItemProperty cmdlet. This command creates or changes the value of the property of a specified item.
The following example shows how to do it with syntax.
# Specify the path to the registry key $registryPath = "HKLM:\Software\SysApp\" # Specify the name of the registry value $propertyName = "AppKey" # Specify the value data $propertyValue = "0100-0fc00-0bd2-002a" # Sets registry value Set-ItemProperty -Path $registryPath -Name $propertyName -Value $propertyValue # Get the registry value Get-ItemProperty -Path $registryPath
Output:
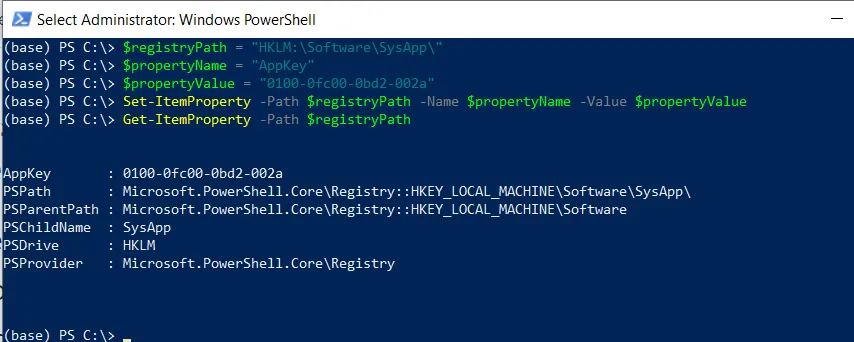
In this example, the Set-ItemProperty cmdlet in PowerShell creates the registry entry. It uses the -Path parameter to specify the path of the registry key, Name to specify the entry name, and Value to specify a value.
The Get-ItemProperty cmdlet is used to see the new registry entry.
You can use the Set-ItemProperty cmdlet to set the registry Dword value for DWord.
The following example shows how to do it with syntax.
# Specify the path to the registry key $registryPath = "HKLM:\Software\SysApp\" # Specify the name of the registry value $propertyName = "SysKey" # Specify the value data $propertyValue = 1 # Sets registry value Set-ItemProperty -Path $registryPath -Name $propertyName -Value $propertyValue -Type DWord # Get registry value Get-ItemProperty -Path $registryPath -Name $propertyName
The output of the above PowerShell script is given below.
SysKey : 1
PSPath : Microsoft.PowerShell.Core\Registry::HKEY_LOCAL_MACHINE\Software\SysApp\
PSParentPath : Microsoft.PowerShell.Core\Registry::HKEY_LOCAL_MACHINE\Software
PSChildName : SysApp
PSDrive : HKLM
PSProvider : Microsoft.PowerShell.Core\Registry
In this example, it sets the registry value to the DWord type.
Conclusion
I hope the above article on setting the registry value using the New-ItemProperty and Set-ItemProperty cmdlets in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.