To delete all files with a specific extension in PowerShell, you can use the Get-ChildItem and Remove-Item cmdlets.
The following method can be used to delete all files with a specific extension in a directory using PowerShell.
Method 1: Delete all files with a specific extension using PowerShell
Get-ChildItem -Path $directoryPath -Filter "*.txt" | Remove-Item
This example retrieves files in the specified directory $directoryPath
with a specified extension (.txt) and pipes them to the Remove-Item
cmdlet, which deletes each file.
Method 2: Delete all files with extension recursively using PowerShell
Get-ChildItem -Path $directoryPath -Filter "*.csv" -Recurse| Remove-Item
In this example, the Get-ChildItem
recursively retrieved all files with an extension (.csv) and pipes to the Remove-Item
cmdlet to delete each of them.
The following example shows how to use these methods.
Delete All Files with a Specific Extension Using PowerShell
Use the Get-ChildItem
cmdlet in PowerShell to retrieve files with a specific extension (.txt) and pass it as input to the Remove-Item
cmdlet to remove each file.
# Specify the directory path $directoryPath = "C:\temp\log\" # Retrieve *.txt files and delete each file Get-ChildItem -Path $directoryPath -Filter "*.txt" | Remove-Item
After running the above PowerShell script, it retrieves all files from the specified folder $directoryPath with .txt extension and pipes it to the Remove-Item cmdlet. The Remove-Item command deletes each file.
Delete All Files with Extension Recursively Using PowerShell
To delete all files with extension recursively, use the Get-ChildItem
cmdlet along with the -Recurse
parameter to retrieve all files from folders and subfolders and pipe it to the Remove-Item
command to delete all files.
The following example shows how to do it with syntax.
# Specify the directory path $directoryPath = "C:\temp\log\" # Retrieve all files recursively with .csv extension Get-ChildItem -Path $directoryPath -Filter "*.csv" -Recurse # Retrieve all files recursively with .csv extension and remove it Get-ChildItem -Path $directoryPath -Filter "*.csv" -Recurse | Remove-Item # Retrieve all files recursively with .csv extension Get-ChildItem -Path $directoryPath -Filter "*.csv" -Recurse
The output of the above PowerShell script deletes all files with a specific extension (.csv) from the specified folder $directoryPath.
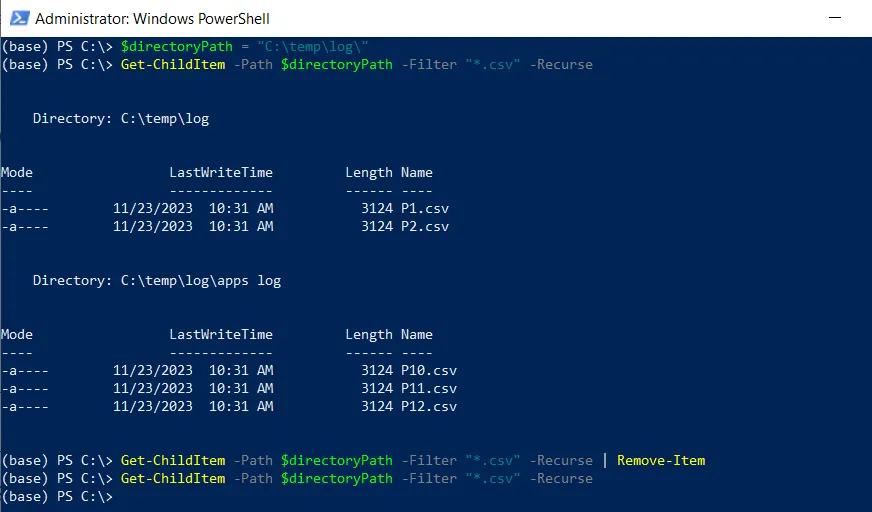
It removes all the files with an extension (.csv) from folders and subfolders.
Conclusion
I hope the above article on deleting all files with an extension using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.