To subtract time from the DateTime variable in PowerShell, you can use any of the below methods.
Method 1: Using the TimeSpan Add() method with a negative value
# define a DateTime object
$date = [DateTime] "2024-04-15 12:30:00 PM"
# Subtract 2 hours from the datetime object
$new_date = $date.Add([TimeSpan]::FromHours(-2))
Write-Output $new_date
This example will output the DateTime object with 2 hours subtracted.
Method 2: Using the Subtraction operator
# define a DateTime object
$date = [DateTime] "2024-04-15 12:30:00 PM"
# Subtract 2 hours from the datetime object
$new_date = $date - [TimeSpan]::FromHours(2)
Write-Output $new_date
This example will output the DateTime object with 2 hours subtracted from a datetime variable.
Method 3: Using the TimeSpan
# define a DateTime object
$date = [DateTime] "2024-04-15 12:30:00 PM"
# Subtract 2 hours 30 minutes from the datetime object
$new_date = $date - [TimeSpan] '2:30'
Write-Output $new_date
This example will output the DateTime object with 2 hours and 30 minutes subtracted from a datetime variable.
The following examples show how you can use these methods.
Subtract Time from a DateTime Variable Using the TimeSpan Add() method
To subtract time in PowerShell from a datetime variable, you can use the TimeSpan struct and its Add() method.
# define a DateTime object $date = [DateTime] "2024-04-15 12:30:00 PM" # Subtract 2 hours from the datetime object $new_date = $date.Add([TimeSpan]::FromHours(-2)) Write-Output $new_date
Output:
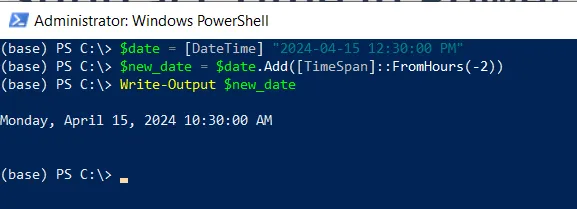
In this PowerShell script, the $date variable stores the datetime. We use the Add() method of TimeSpan struct to subtract 2 hours using the FromHours() method.
Finally, we output the DateTime object with 2 hours subtracted from a datetime variable $date.
Subtract Time Using the Subtraction Operator
You can use the Subtraction operator to subtract the specified amount of time ( 2 hours) from the given datetime object.
# define a DateTime object $date = [DateTime] "2024-04-15 12:30:00 PM" # Subtract 2 hours from the datetime object $new_date = $date - [TimeSpan]::FromHours(2) Write-Output $new_date
Output:
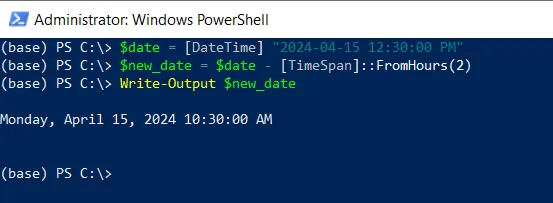
This PowerShell script uses the subtract operator (–) to subtract 2 hrs from the datetime variable and output to the console using the Write-Output cmdlet.
Subtract Time from DateTime Variable Using the TimeSpan
Another way to subtract time from a datetime variable is to use the TimeSpan struct and specify the amount of time to subtract.
# define a DateTime object $date = [DateTime] "2024-04-15 12:30:00 PM" # Subtract 2 hours 30 minutes from the datetime object $new_date = $date - [TimeSpan] '2:30' Write-Output $new_date
Output:
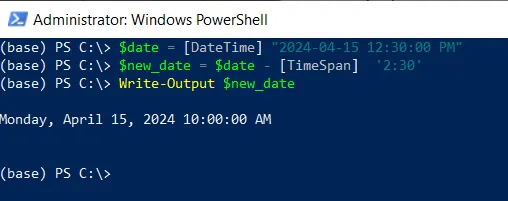
This example outputs the date time object by subtracting 2 hours and 30 minutes from the $date variable.
Conclusion
I hope the above article on subtracting time from a datetime variable in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.