You can compare dates in PowerShell using multiple ways.
The following methods show how to compare dates with syntax.
Method 1: Compare dates using the comparison operator
#create two date objects
$date1 = [DateTime] "2024-04-02"
$date2 = [DateTime] "2024-04-15
# compare dates
if($date1 -lt $date2 {
Write-Output "Date 1 ($date1) is earlier than Date 2 ($date2)."
}
elseif ($date1 -eq $date2) {
Write-Output "Date 1 ($date1) is the same as Date 2 ($date2)."
}
else {
Write-Output "Date 1 ($date1) is later than Date 2 ($date2)."
}
This example compares two datetime objects using the comparison operators to check if $date1 is earlier, the same, or later than $date2.
Method 2: Compare dates using the CompareTo() method
#create two date objects
$date1 = [DateTime] "2024-04-17"
$date2 = [DateTime] "2024-04-15"
#compare two dates
$result = $date1.CompareTo($date2)
if ($result -eq -1) {
Write-Output "$date1 is earlier than $date2."
}
elseif ($result -eq 0) {
Write-Output "$date1 is the same as $date2."
}
else {
Write-Output "$date1 is later than $date2."
}
This example will compare dates using the CompareTo() method.
The following example shows how you can use these methods.
Compare Dates in PowerShell Using Comparison Operator
To compare dates in PowerShell, you can use the comparison operator (-lt, -le, -gt, -ge, -eq, -ne) with the dates to be compared.
#create two date objects $date1 = [DateTime] "2024-04-02" $date2 = [DateTime] "2024-04-15" # compare dates if($date1 -lt $date2) { Write-Output "$date1 is earlier than $date2" } elseif ($date1 -eq $date2) { Write-Output "$date1 is later than $date2" } else { Write-Output "$date1 is equal to $date2" }
Output:
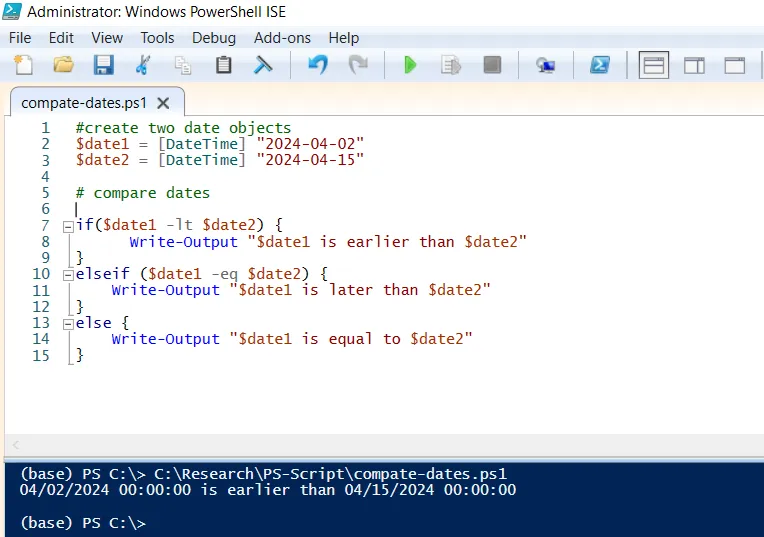
In this script, we have created two datetime objects $date1 and $date2, and assigned a date to them. The comparison operators are used to check if the $date1 is earlier, the same, or later than the $date2.
Finally, the Write-Output cmdlet outputs the result on the console.
Compare Dates Using CompareTo() Method
Another way to compare dates in PowerShell is by using the CompareTo() method of the DateTime struct. This method returns -1, 0, or 1 depending on whether the date is earlier, same, or later than the specified date.
#create two date objects $date1 = [DateTime] "2024-04-17" $date2 = [DateTime] "2024-04-15" #compare two dates $result = $date1.CompareTo($date2) if ($result -eq -1) { Write-Output "$date1 is earlier than $date2." } elseif ($result -eq 0) { Write-Output "$date1 is the same as $date2." } else { Write-Output "$date1 is later than $date2." }
Output:
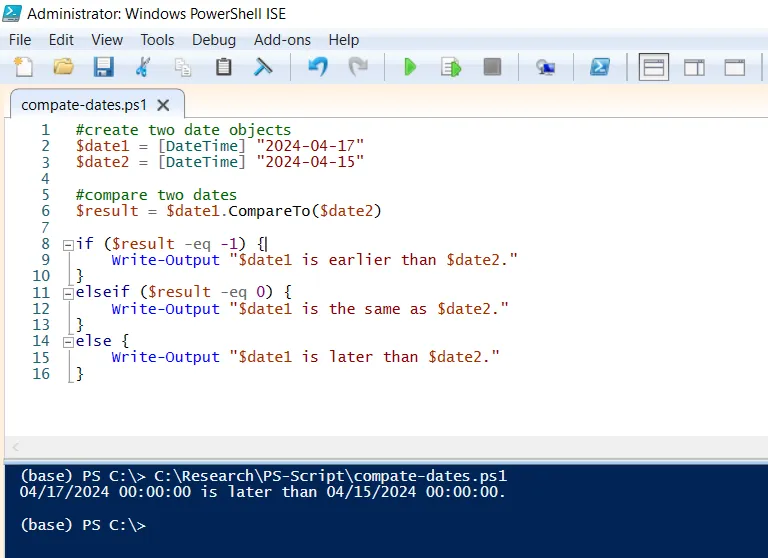
In this script, the $date1 and $date2 variables store two dates. We used the CompareTo method to check $date1 with $date2. It returns -1, 0, or 1 value.
Finally, we used the if else statement to check the $result value and print the output message using the Write-Out cmdlet.
Conclusion
I hope the above article on how to compare two dates in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.