To get the difference between two dates in PowerShell, you can subtract one date from another, and then use the TotalDays property of the TimeSpan object to calculate the difference in days.
The following method shows how you can do it with syntax.
Method 1: Get the date difference in Days
#Create two DateTime objects
$date1 = [DateTime] "2024-04-15"
$date2 = Get-Date
# Calculates the difference between two dates in days
$dateDifference = ($date2 -$date1).TotalDays
Write-Output "The difference between the two dates is: $dateDifference days."
This example will output the date difference between the two dates in days.
The following example shows how to use this method.
Get the Date Difference in Days in PowerShell
Suppose we have two dates $date1 and $date2. To find the date difference in days between these two dates, follow the below syntax.
#Create two DateTime objects $date1 = [DateTime] "2024-04-15" $date2 = Get-Date # Find the date difference between two dates in days $dateDifference = ($date2 -$date1).TotalDays Write-Output "The date difference between the two dates is: $dateDifference days."
Output:
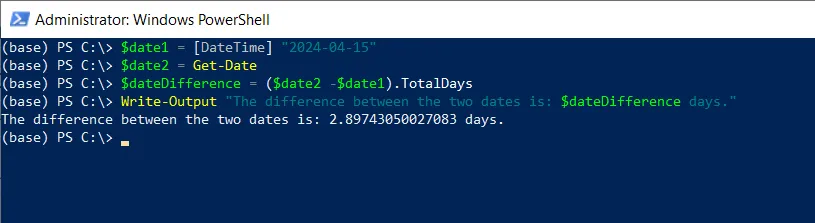
In this script, the $date1 and $date2 variables are set to specific dates. The $dateDifference calculates the difference between two dates in days using the TotalDays property.
Finally, it outputs the difference between the two dates in days using the Write-Output cmdlet.
You can also get the date difference in a different time unit, like hours, minutes, or seconds.
# Calculates the difference in hours $hourDifference = ($date2 -$date1).TotalHours Write-Output "The difference between the two dates is: $hourDifference hours." # Calculates the difference in minutes $minutesDifference = ($date2 -$date1).TotalMinutes Write-Output "The difference between the two dates is: $minutesDifference minutes." # Calculates the difference in seconds $secondsDifference = ($date2 -$date1).TotalSeconds Write-Output "The difference between the two dates is: $secondsDifference seconds."
Output:
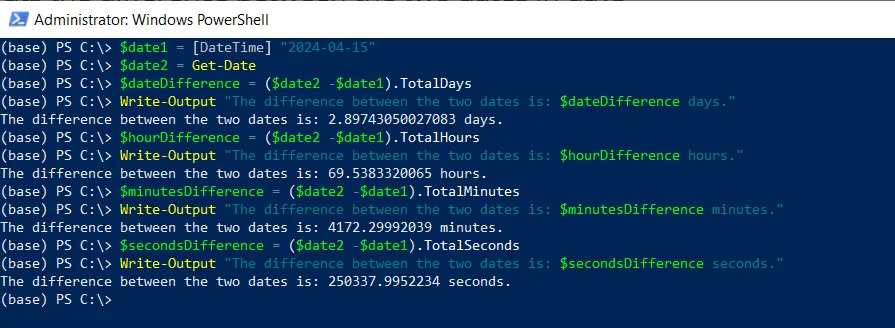
This example outputs the date difference in dates in hours, minutes, and seconds.
Conclusion
I hope the above article on calculating the difference in days in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.