PowerShell offers several ways to join multiple strings together.
You can use the following methods to concatenate strings in PowerShell:
Method 1: Using the + Operator
$fullName = $firstName + $lastName
Method 2: Using the Join Operator
$fullName = $firstName, $lastName -join " "
Method 3: Using the Format Operator
$welcomeMsg = "Hello, {0}!" -f $firstName
Method 4: Using the String Interpolation
$welcomeMsg = "Hello, $firstName!"
All of the above methods are used to combine the variables.
The following examples demonstrate the practical application of each method for string concatenation.
Concatenate Strings in PowerShell Using Plus Operator
The simplest method to concatenate strings in PowerShell is using the plus (+) operator in between the string variables.
For example, the following PowerShell script concatenates two strings with space as a separator.
$firstName = "Aron" $lastName = "Smith" $fullName = $firstName + " " + $lastName Write-Host $fullName
The following output displays the concatenation of strings together with space as a separator in PowerShell.
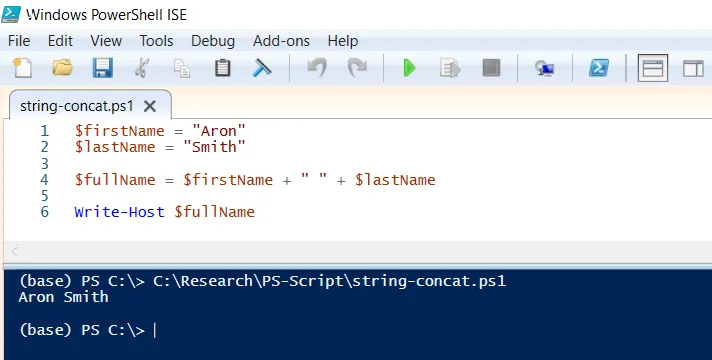
Concatenate Strings in PowerShell Using Join Operator
We can use the join operator followed by a separator to concatenate strings together in PowerShell.
For example, the following PowerShell script joins the two strings together with a space as a separator.
$firstName = "Aron" $lastName = "Smith" $fullName = $firstName, $lastName -join " "
The output of the above PowerShell script shows two strings combined with a single space in between them.
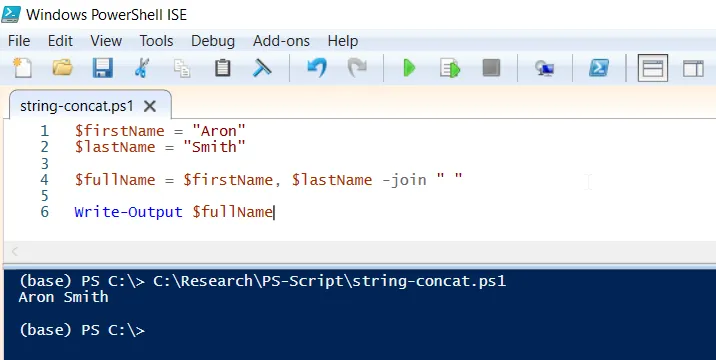
You can also use a different separator after the -join operator to concatenate strings.
Concatenate Strings in PowerShell Using Format Operator
Another way to concatenate strings and variables together in PowerShell is by using the format operator -f.
We can use the following PowerShell script that concatenates strings and variables together by using the format operator.
$firstName = "Adam" $welcomeMsg = "Hello, {0}!" -f $firstName Write-Output $welcomeMsg
The following output shows how to concatenate strings and variables using the format operator. The -f operator is used to embed values into a string.
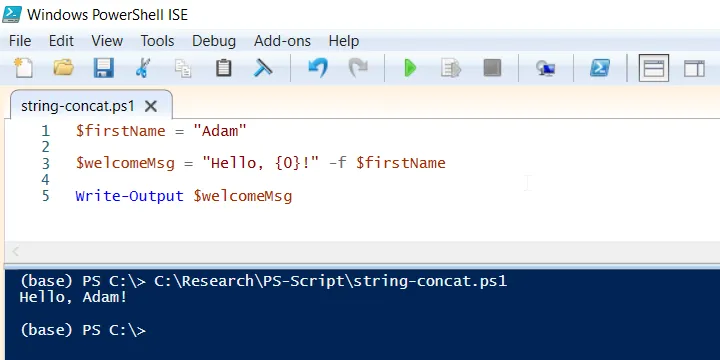
Concatenate Strings in PowerShell Using String Interpolation
PowerShell supports string interpolation that allows us to embed expressions within a string.
The following examples show the concatenation of strings in PowerShell.
$firstName = "Adam" $welcomeMsg = "Hello, $firstName!" Write-Output $welcomeMsg
The output of the above PowerShell script ended a variable within a string using string interpolation.
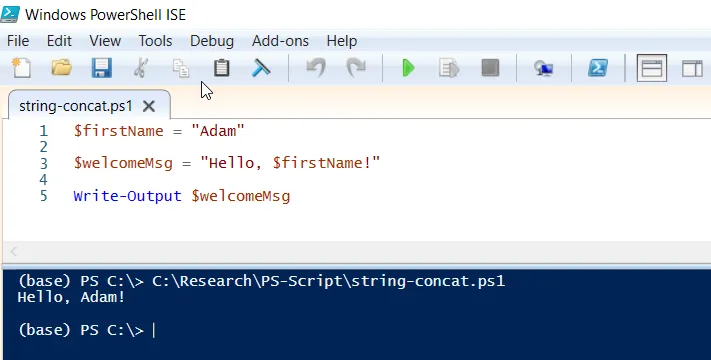
$firstName
variable is embedded within the string assigned to the $welcomeMsg
variable. String interpolation is a method of constructing a new string by replacing placeholders ( in this $firstName
) with provided values.
PowerShell parses the $firstName
variable and replaces it with the value “Adam” before outputting the final string.
Conclusion
I hope the above article concatenation of strings using multiple ways such as plus sign (+) operator, join operator, format operator, and string interpolation is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.