In PowerShell, there are several ways to replace text in string in PowerShell.
Method 1: Using the Replace() method
$new_string = $original_string.Replace("old_text","new_text")
Method 2: Using the -replace operator
$new_string = $original_string -replace "old_text", "new_text"
Method 3: Using the -creplace operator
$new_string = $original_string -creplace "old_text", "new_text"
Method 4: Using the -ireplace operator
$new_string = $original_string -ireplace "old_text", "new_text"
Method 5: Using the [regex]::Replace() method
$new_string = [regex]::Replace($original_string, $pattern, $replace_string)
All of these methods replace text in string stored in the variable $original_string
.
The following examples demonstrate how to use these methods in practical application.
Replace Text in String in PowerShell by Using Replace() Method
The simplest method to replace text in a string in PowerShell is using the Replace() method.
This example replaces the “sample” text with “demo” in a string variable named $original_string
and stores the output in the $new_string
variable.
$original_string = "Hello, this is a sample script" $new_string = $original_string.Replace("sample","demo") Write-Output $new_string
The output of the above PowerShell script shows how to replace a text in a string.
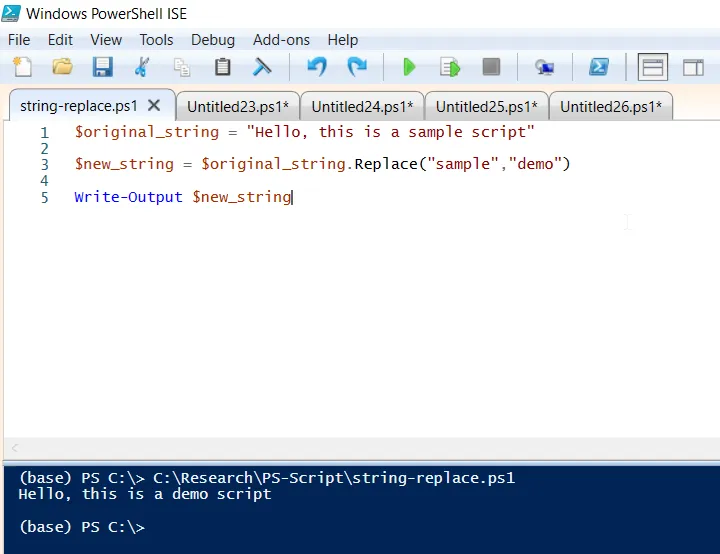
It replaces “sample” text with “demo” text in a string variable.
Replace Text in String in PowerShell by Using -replace Operator
Another way to replace text in a string in PowerShell is using the -replace operator.
This example replaces the “sample” text with the “demo” in a string variable named $original_string
and stores the output in the $new_string
variable.
$original_string = "Hello, this is a sample script" $new_string = $original_string -replace "sample", "demo" Write-Output $new_string
The output of the above PowerShell script shows how to replace a text in a string.
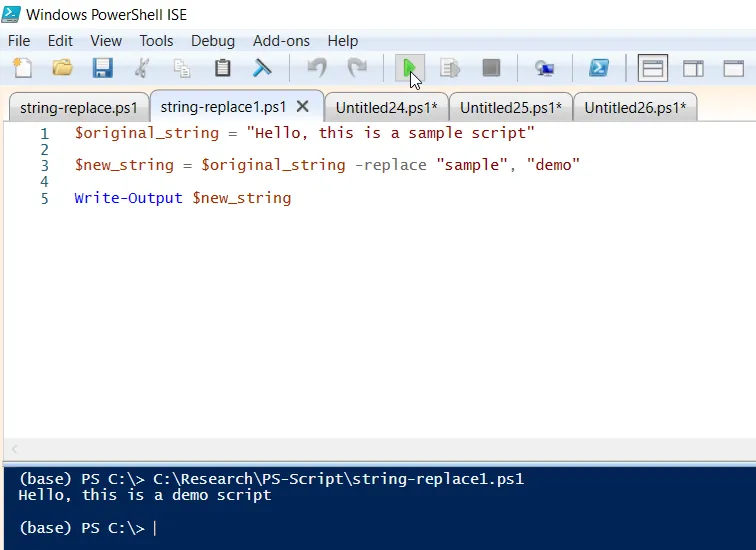
It replaces “sample” text with “demo” text in a string variable.
Replace Text in String in PowerShell by Using -creplace() Operator
The -creplace
operator is similar to -replace
operator, but it is case-sensitive and used to replace text in a string.
The following example demonstrates how we can use it to replace the text “Sample” with “Demo” in a string variable $original_string
.
$original_string = "Hello, this is a Sample script" $new_string = $original_string -creplace "Sample", "Demo" Write-Output $new_string
The output of the above PowerShell script is given below.
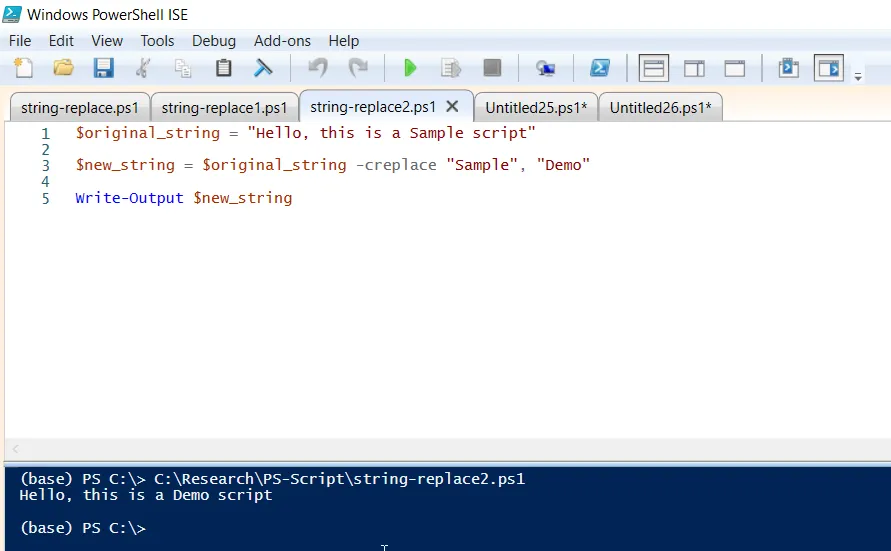
The -creplace
operator replaces “Sample” text with “Demo” text in a string variable. It is case-sensitive.
Replace Text in String in PowerShell by Using -ireplace Operator
The -ireplace
operator is similar to -replace
operator, but it is case-insensitive and used to replace text in a string.
Here is an example that demonstrates how we can use it to replace the text “sample” with “Demo” in a string variable $original_string
.
$original_string = "Hello, this is a Sample script" $new_string = $original_string -ireplace "sample", "Demo" Write-Output $new_string
The following output shows how to use the -ireplace
operator to replace the text “sample” with a new text “Demo” in the string.
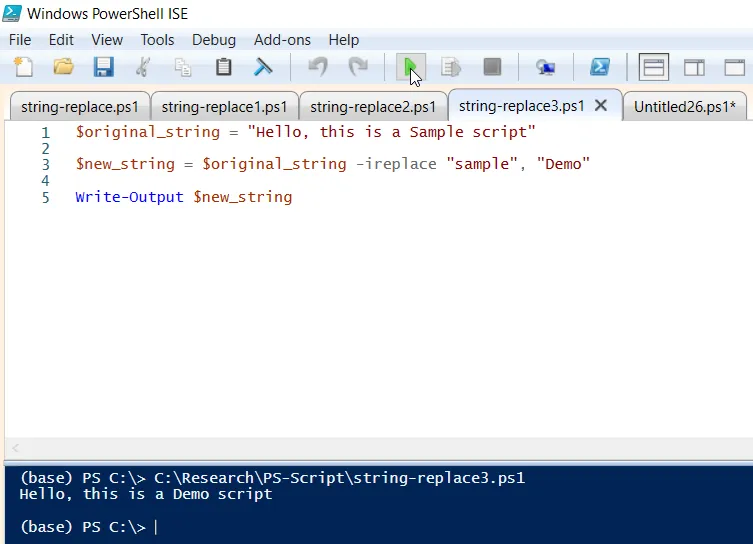
The -ireplace operator replaces “Sample” text with “Demo” text in a string variable. It is case-insensitive.
Replace Text in String in PowerShell by Using [regex]::Replace() Method
Another way to replace a specific text in a string in PowerShell is by using the .NET Regular Expression classes directly. We can use the [regex]::Replace() method.
Here’s a sample practical example to replace “sample” with “Demo” in a string variable named $original_string
.
$original_string = "Hello, this is a sample script" $pattern = "\bsample\b" $replace_string = "Demo" $new_string = [regex]::Replace($original_string, $pattern, $replace_string) Write-Output $new_string
The output of the above PowerShell script is given below.
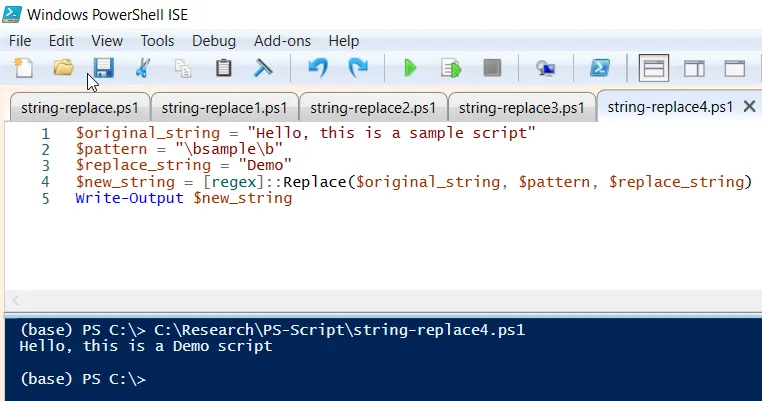
The $pattern
variable is set to “\bsample\b“. Here, \b
represents a word boundary, ensuring that a “sample” is matched as a whole word.
The Replace()
method from [regex]
class is used to replace all occurrences of the pattern with the replacement string $replace_string
.
Finally, the modified string is output using the Write-Output cmdlet
Conclusion
I hope the above article on how to replace text in a string in PowerShell using several ways such as Replace() method, -replace operator, -creplace, -ireplace operator and regex class Replace() method is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.