In PowerShell, there are several ways to convert an object to a string, including the ToString() method, the Out-String cmdlet, and the string interpolation.
The following methods show how you can do it with syntax.
Method 1: Using ToString() method
# define datetime object
$date = [DateTime] "2024-04-16 12:00:00 PM"
# convert the object to a string
$stringDate = $date.ToString()
# write the output
Write-Output "String representation of datetime object is : $stringDate"
This example will write the string representation of a datetime object.
Method 2: Using the Out-String cmdlet
# define a service object
$service = Get-Service -Name "Docker Desktop Service"
# convert the object to a string
$stringService = $service | Out-String
# output the string
Write-Output "String representation of service object is : $stringService"
This example will output the string representation of service object.
Method 3: Using the string interpolation
# define an object
$employee = [PSCustomObject] @{
Name = "Adam"
Age = 27
}
# convert the object to a string
$stringEmp = "$($employee.Name) is $($employee.Age) years old."
# Write the output
Write-Output "String representation of employee object is : $stringEmp"
This example will create an employee object using PSCustomObject and convert it into a string.
The following example shows how you can use each one of the methods.
Convert Object to String Using ToString() Method
You can use the ToString() method to convert an object to a string in PowerShell.
# define datetime object $date = [DateTime] "2024-04-16 12:00:00 PM" # convert the object to a string $stringDate = $date.ToString() # write the output Write-Output "String representation of datetime object is : $stringDate"
Output:
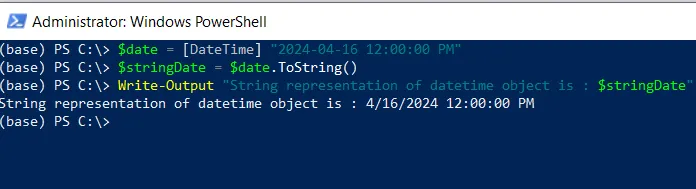
In this script, we defined a datetime object and stored it in the $date variable. We then use the ToString() method over the datetime object to convert an object into a string.
Finally, the Write-Output cmdlet outputs the string representation of a datetime object.
Convert Object to String Using Out-String Cmdlet
The easy way of converting an object to a string is to use Out-String cmdlet.
# define a service object $service = Get-Service -Name "Docker Desktop Service" # convert the object to a string $stringService = $service | Out-String # output the string Write-Output "String representation of service object is : $stringService"
Output:
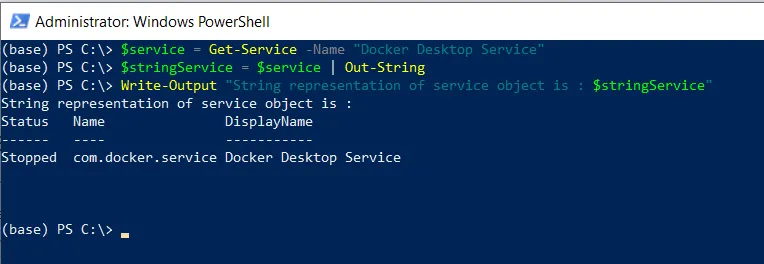
In this script, we defined a service object using the Get-Service cmdlet and assigned it to the $service variable. We then pipe the $service object to the Out-String cmdlet to convert it into a string and store it in the $stringService variable
Finally, we use the Write-Output cmdlet to print the string representation of the service object.
Convert Object to String Using String Interpolation
Another way to convert an object to a string is to use the string interpolation in PowerShell.
# define an object $employee = [PSCustomObject] @{ Name = "Adam" Age = 27 } # convert the object to a string $stringEmp = "$($employee.Name) is $($employee.Age) years old." # Write the output Write-Output "String representation of employee object is : $stringEmp"
Output:
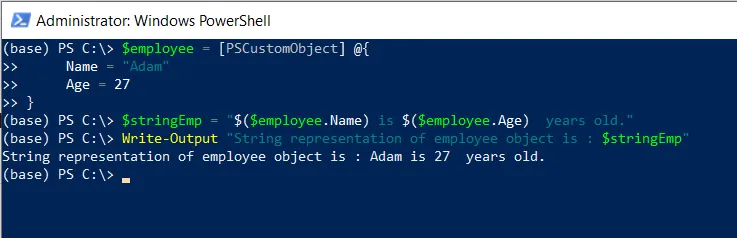
In this script, we created a $employee object using the PSCustomObject. We then use the string interpolation to convert an object to a string.
Finally, we use the Write-Output cmdlet in PowerShell to output the string representation of the employee object.
Conclusion
I hope the above article on converting an object to a string in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.