To delete files matching patterns in PowerShell, you can use the Get-ChildItem and Remove-Item cmdlets.
The following methods can be used to retrieve all files that match the patterns and delete all of them.
Method 1: Delete file matching pattern in PowerShell
# specify the directory path
$directoryPath = "C:\temp\log\"
# specify the pattern
$pattern = "*sys_*"
# Retrieve file that matches pattern and remove them
Get-ChildItem -Path $directoryPath -Filter $pattern -File -Recurse -Force | Remove-Item -Force
This example deletes files that match the pattern specified in the $pattern.
Method 2: Delete all files matching the pattern in PowerShell
# specify the directory path
$directoryPath = "C:\temp\log\"
# specify the pattern
$pattern = "*sys_*"
# Retrieve file that matches pattern and remove them
Get-ChildItem -Path $directoryPath | Where {$_.Name -match "sys_*"} | Remove-Item -Force
This example removes all files matching pattern $pattern.
The following examples show how to use these methods.
Delete Files Matching Pattern in PowerShell
To delete all files matching a pattern in PowerShell, use the Get-ChildItem cmdlet to retrieve the list of files that match the patterns and pipe them to the Remove-Item cmdlet for deleting all of the files that match the pattern.
The following PowerShell script shows how to do it with syntax.
# specify the directory path $directoryPath = "C:\temp\log\" # specify the pattern $pattern = "*sys_*" # Retrieve file that matches pattern and remove them Get-ChildItem -Path $directoryPath -Filter $pattern -File -Recurse -Force | Remove-Item -Force
Output:
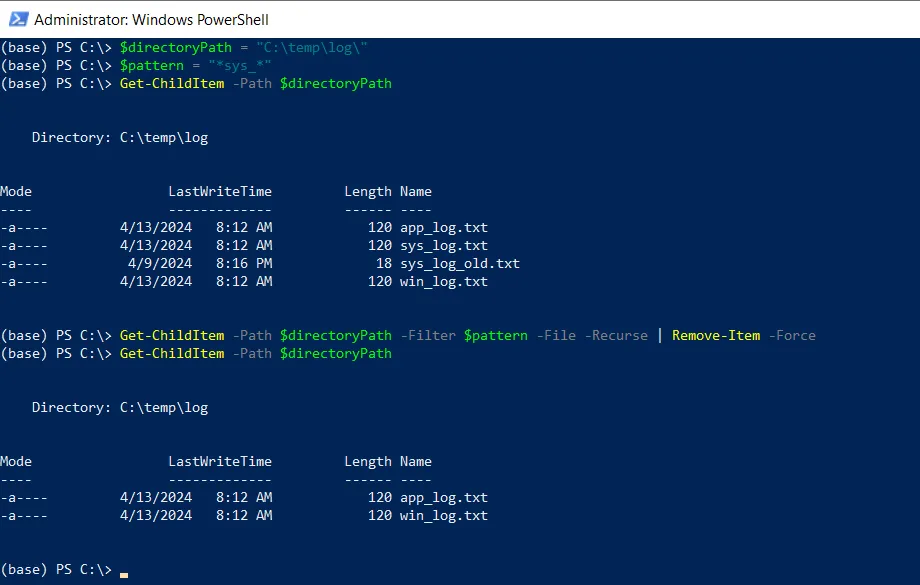
In this example, the $directoryPath variable stores the directory path. The $pattern variable stores the pattern “*sys_*“.
The Get-ChildItem cmdlet retrieves the list of files from the specified $directoryPath that matches the pattern $pattern. It matches the files having “*sys_*” in their file name, filters those files, and pipes them to the Remove-Item cmdlet.
The Remove-Item cmdlet deletes all files matching a certain pattern.
Delete All files Matching the Pattern in the PowerShell
Another way to delete all files matching the pattern in PowerShell is using the Get-ChildItem cmdlet along with the Where condition to check the matching pattern and filter the files for deletion by the Remove-Item cmdlet.
The following PowerShell script shows how to do it with syntax.
# specify the directory path $directoryPath = "C:\temp\log\" # Retrieve file that matches pattern and remove them Get-ChildItem -Path $directoryPath | Where {$_.Name -match "*sys_*"} | Remove-Item -Force
Output:
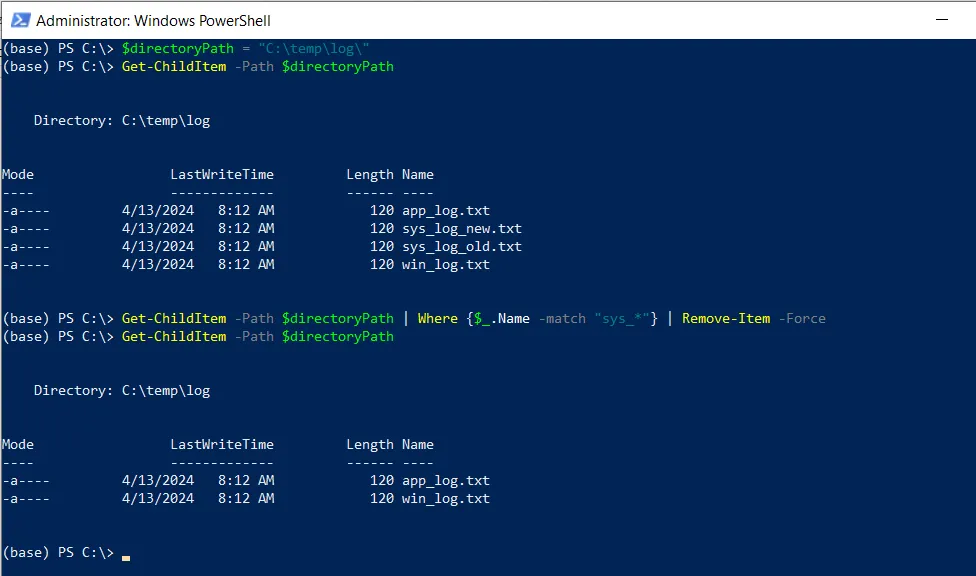
In the above PowerShell script, the Get-ChildItem cmdlet retrieves the list of files from the specified directory path $directoryPath and pipes them to the Where condition.
The Where condition checks if the name of the file matches with the specified patterns “*sys_*” and pipes the files to the Remove-Item cmdlet. The Remove-Item command deletes all files that contain “*sys*” in the file name.
Conclusion
I hope the above article on how to delete files matching patterns in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.