To list files in a directory and save them to a text file in PowerShell, you can use the Get-ChildItem cmdlet to retrieve the files and pipe them to Out-File cmdlet to output to a text file.
The following methods show how to use the syntax to list files and save them to a text file or csv file.
Method 1: List files in a directory to a text file in PowerShell
$directoryPath = "C:\temp\log\"
$outFilePath = "C:\temp\file_list.txt"
Get-ChildItem -Path $directoryPath -File -Force | Select-Object FullName | Out-File -FilePath $outFilePath
This example will list all files in a directory to a text file using the Get-ChildItem and Out-File cmdlets.
Method 2: List files in a directory to a csv file in PowerShell
$directoryPath = "C:\temp\log\"
$outFilePath = "C:\temp\file_list.csv"
Get-ChildItem -Path $directoryPath -File -Force | Select-Object FullName > $outFilePath
This example retrieves the list of files in a directory using the Get-ChildItem and saves them to a csv file using the output redirection operator (>).
Method 3: List all files in the directory and subdirectories to csv file in PowerShell
$directoryPath = "C:\temp\log\"
$outFilePath = "C:\temp\file_list.csv"
Get-ChildItem -Path $directoryPath -File -Recurse -Force | Select-Object FullName > $outFilePath
This example retrieves the list of files in the directory and subdirectories using the Get-ChildItem cmdlet and its -Recurse parameter. It saves them to a csv file using the output redirection operator (>).
The following examples show how to use these methods.
List Files in a Directory to Text File in PowerShell
To list files in a directory to a text file in PowerShell, you can use the Get-ChildItem that retrieves the files from the specified directory and Out-File cmdlet to save file names to a text file.
The following example shows how to do it with syntax.
# Specify the directory path $directoryPath = "C:\temp\log\" # Specify the text file path where you want to save list of all file names $outFilePath = "C:\temp\file_list.txt" # Retrieve list of all file names and save them to text file Get-ChildItem -Path $directoryPath -File -Force | Select-Object FullName | Out-File -FilePath $outFilePath # read the content of the text file Get-Content -Path $outFilePath
Output:
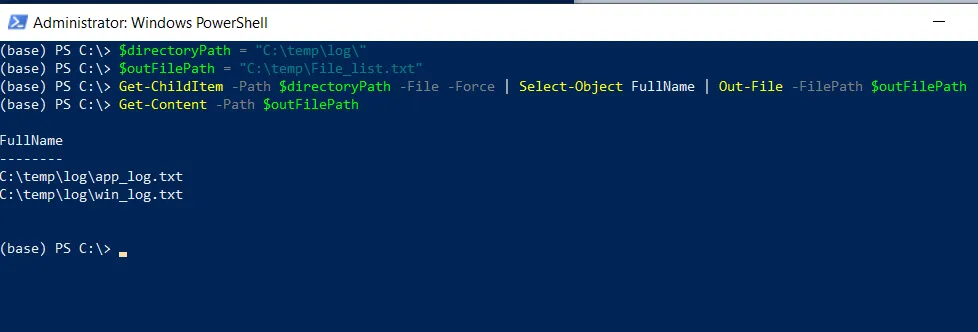
In the above PowerShell script, the $directoryPath variable holds the path to the directory having files. $outFilePath variable stores the text file name where you want to save the list of all file names.
The Get-ChildItem cmdlet retrieves the list of all files from the specified directory $directoryPath and pipes them to the Select-Object cmdlet to select the file’s full name.
Finally, a list of all file names is output to a text file named “File_list.txt” using the Out-File cmdlet in PowerShell.
If we try to read the contents of the text file named “File_list.txt” using the Get-Content cmdlet, it displays the list of file names with their full file path.
List Files in a Directory to a CSV file in PowerShell
Another way to list files in a directory to a csv file in PowerShell is by using the Get-ChildItem cmdlet to retrieve all files from the directory and use the output redirection operator (>) to output file names to the csv file.
The following example shows how to use it with syntax.
# Specify the directory path $directoryPath = "C:\temp\log\" # Specify the text file path where you want to save list of all file names $outFilePath = "C:\temp\file_list.csv" # Retrieve list of all file names and save them to csv sile Get-ChildItem -Path $directoryPath -File -Force | Select-Object FullName > $outFilePath # read the content of csv file Get-Content -Path $outFilePath
Output:
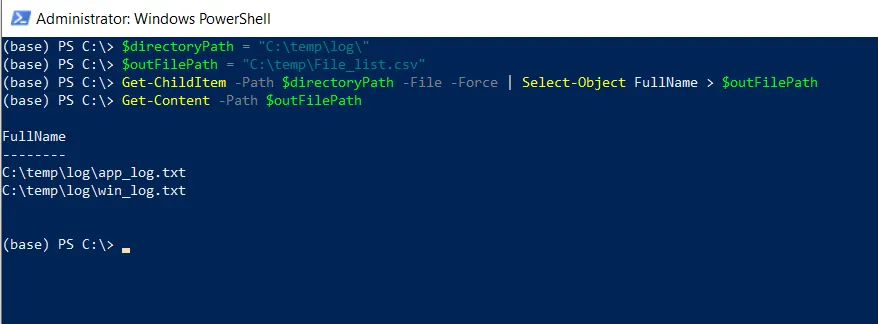
In the above PowerShell script, the $directoryPath variable holds the path to the directory path having files. The $outFilePath variable stores the csv file path where you want to save a list of all file names.
The Get-ChildItem cmdlet retrieves the list of all files from the specified directory $directoryPath and pipes them to the Select-Object cmdlet to select the file’s full name.
Finally, the list of file names in the directory is output to a csv file named “File_list.csv” using the output redirection operator (>).
List all files in the directory and subdirectories to csv file in PowerShell
To list all files in the directory and subdirectories to csv file in PowerShell, you can use the Get-ChildItem cmdlet with its -Recurse parameter and output to a csv file using the output redirection operator (>).
The following example shows how to do it with syntax.
# Specify the directory path $directoryPath = "C:\temp\log\" # Specify the text file path where you want to save list of all file names $outFilePath = "C:\temp\file_list.csv" # Retrieve list of all file names and save them to csv sile Get-ChildItem -Path $directoryPath -File -Recurse -Force | Select-Object FullName > $outFilePath # read the content of csv file Get-Content -Path $outFilePath
Output:
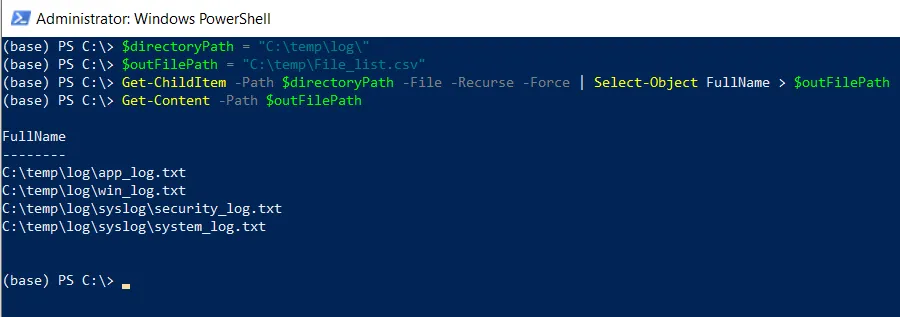
In the above PowerShell script, the $directoryPath variable holds the path to the directory path having files. The $outFilePath variable stores the csv file path where you want to save a list of all file names.
The Get-ChildItem cmdlet retrieves the list of all files from the specified directory and subdirectories using its Recurse parameter. It pipes them to the Select-Object cmdlet to select the file’s full name.
Finally, the list of file names in the directory is output to a csv file named “File_list.csv” using the output redirection operator (>).
Coclusion
I hope the above article on listing all files in a directory to text file using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.