To find files modified between dates in PowerShell, you can use the Get-ChildItem cmdlet to retrieve the files from a directory and filter based on the LastWriteTime property of the file for a given date range.
The following method shows how to do it with syntax.
Method 1: Find files between the date range in PowerShell
# specify the folder path
$folderPath = "C:\temp\"
# specify the dates
$date1 = (Get-Date).AddDays(-15)
$date2 = (Get-Date)
# retrieve file and filter based on the last write time of the file
Get-ChildItem -Path $folderPath | Where-Object {$_.LastWriteTime -gt $date1 -and $_.LastWriteTime -lt $date2}
This example will return the files modified between the date range.
The following example shows how to use this method.
Find Files Modified Between Dates in PowerShell
You can use the Get-ChildItem cmdlet to retrieve the files from the directory and filter them based on the given date range to check if the file was modified between dates.
# specify the folder path $folderPath = "C:\temp\" # specify the dates $date1 = (Get-Date).AddDays(-15) $date2 = (Get-Date) # retrieve file and filter based on the last write time of the file Get-ChildItem -Path $folderPath | Where-Object {$_.LastWriteTime -gt $date1 -and $_.LastWriteTime -lt $date2}
Output:
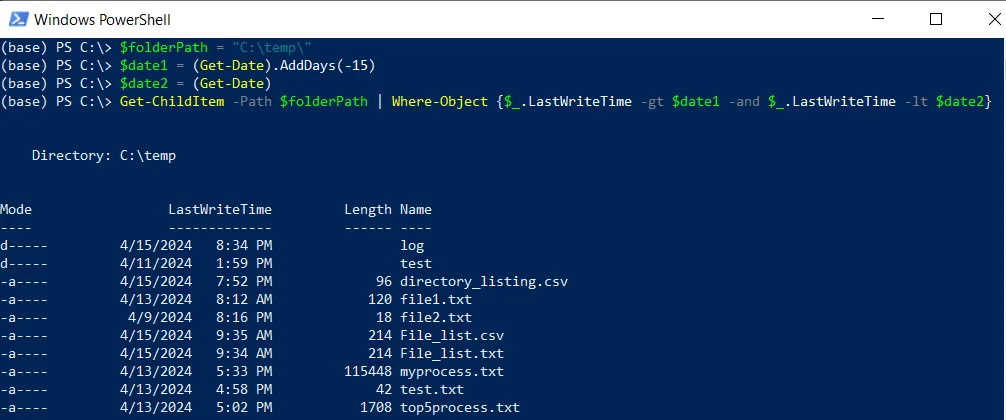
After executing this PowerShell script, the Get-ChildItem lists all files from the specified directory $folderPath and pipes them to the Where-Object command to check if the LastWriteTime of the file is between the provided dates range.
The output of the PowerShell script lists files modified between dates.
Conclusion
I hope the above article on finding files modified between date ranges in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.