To get the filename without the extension from a full file path in PowerShell, you can use the Split-Path command to extract the filename and then use the Split() method or System.IO.Path .NET class to remove the extension.
The following methods can be used to get a filename without extension in PowerShell.
Method 1: Using the Split-Path and Split() method
# specify the full file path
$filePath = "C:\temp\log\my_log.txt"
# Get the filename from the path
$fileName = Split-Path -Path $filePath -Leaf
# Remove the extension from the filename
$fileNameWithoutExtension = $fileName.Split(".")[0]
# Write the filename without extension
Write-Output $fileNameWithoutExtension
This example will extract the filename from the full file path without extension using the Split-Path cmdlet and Split() method.
Method 2: Using the [System.IO.Path] .NET class
# specify the full file path
$filePath = "C:\temp\log\my_log.txt"
# Get the filename from the path
$fileName = Split-Path -Path $filePath -Leaf
# Remove the extension from the filename
$fileNameWithoutExtension = [System.IO.Path]::GetFileNameWithoutExtension($fileName)
# Write the filename without extension
Write-Output $fileNameWithoutExtension
This example will get the filename without an extension from a full file path using the .NET class.
The following examples show how to use these methods.
Get FileName without Extension Using Split-Path Cmdlet and Split() Method
You can use the Split-Path cmdlet to extract the filename from the full file path and then use the Split() method to get the filename without an extension.
# specify the full file path $filePath = "C:\temp\log\my_log.txt" # Get the filename from the path $fileName = Split-Path -Path $filePath -Leaf # Remove the extension from the filename $fileNameWithoutExtension = $fileName.Split(".")[0] # Write the filename without extension Write-Output $fileNameWithoutExtension
Output:
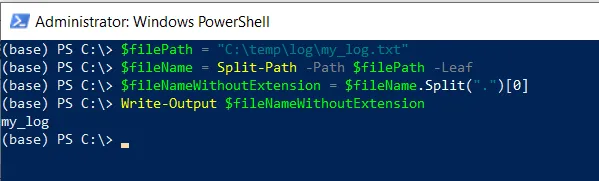
In this script, the $filePath variable stores the full file path. The Split-Path cmdlet uses the -Path and -Leaf parameters to extract the filename from the full file path and stores it in the $fileName variable.
Finally, it uses the Split() method over the $fileName variable to get the filename without an extension and output to the console using the Write-Output cmdlet.
After executing the script, it displays the file name without an extension “my_log” onto the console.
Get FileName without Extension Using System.IO.Path class
You can use the [System.IO.Path] .NET class to get the file name without an extension. It uses the GetFileNameWithoutExtension() method to extract the filename.
# specify the full file path $filePath = "C:\temp\log\my_log.txt" # Get the filename from the path $fileName = Split-Path -Path $filePath -Leaf # Remove the extension from the filename $fileNameWithoutExtension = [System.IO.Path]::GetFileNameWithoutExtension($fileName) # Write the filename without extension Write-Output $fileNameWithoutExtension
Output:
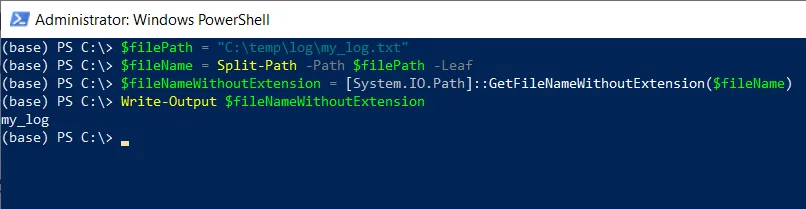
In this script, the $filePath variable stores the full file path. The Split-Path command uses the -Path and -Leaf parameters to extract the filename from the full file path and store it in the $fileName variable.
Finally, it uses the GetFileNameWithoutExtension() method of the [System.IO.Path] class to get the filename and output to the console using the Write-Output command.
After executing the script, it displays the filename without an extension “my_log” onto the console.
Conclusion
I hope the above article on getting the filename without an extension using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.